JavaScript: Check a string is in Kebab case
JavaScript String: Exercise-54 with Solution
Kebab case: "the-quick-brown-fox-jumps-over-the-lazy-dog"
Similar to snake case, above, except hyphens rather than underscores are used to replace spaces. It is also known as spinal case, param case, Lisp case in reference to the Lisp programming language, or dash case (or illustratively as kebab-case).
Write a JavaScript function to check a given string is in Kebab case or not.
Test Data:
('j') -> false
('Java-Exercises') -> true
('JavaScript-Exercises') -> true
('javascript_exercises') -> false
(12356) -> "It must be a string."
Sample Solution:
JavaScript Code:
// Define a function called 'test' that takes one parameter 'text'.
const test = (text) => {
// Check if the type of the 'text' parameter is not a string.
if (typeof text !== 'string') {
// If it's not a string, return the message "It must be a string."
return 'It must be a string.';
}
// Define a regular expression pattern that matches strings containing a hyphen between words.
const pattern = /(\w+)-(\w)([\w-]*)/;
// Return true if the input text matches the pattern and does not contain an underscore, otherwise return false.
return pattern.test(text) && !text.includes('_');
}
// Test the 'test' function with different inputs and log the results.
console.log(test('j'));
console.log(test('Java-Exercises'));
console.log(test('JavaScript-Exercises'));
console.log(test('javascript_exercises'));
console.log(test(12356));
Output:
false true true false It must be a string.
Explanation:
The above JavaScript code defines a function "test()" that checks whether a given input text meets certain criteria:
- It ensures that the input parameter 'text' is a string. If not, it returns the message "It must be a string."
- It defines a regular expression pattern that matches strings containing a hyphen between words. The pattern is /(\w+)-(\w)([\w-]*)/.
- It returns 'true' if the input text matches the defined pattern and does not contain an underscore (_), indicating that it follows the specified format. Otherwise, it returns 'false'.
Flowchart:
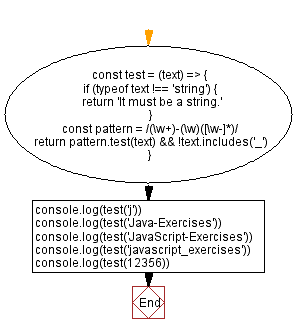
Live Demo:
See the Pen javascript-string-exercise-54 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Check a string is in Flat case.
Next: Check a string is a Pangram or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-string-exercise-54.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics