JavaScript: Check a string is in Flat case
JavaScript String: Exercise-53 with Solution
Check Flat Case
Flat case: As the name implies, flatcase refers to the use of lowercase letters, with no spaces between words.
Write a JavaScript function to check a given string is in Flat case or not.
Test Data:
('j') -> true
('java exercises') -> false
('JavaScriptExercises') -> false
('javascriptexercises') -> true
(12356) -> "It must be a string."
Sample Solution:
JavaScript Code:
/**
* Define a function named test which takes a single parameter 'text'.
* @param {string} text - The input text to be tested.
* @returns {boolean|string} - Returns true if the input text contains only lowercase letters, otherwise returns a string message.
*/
const test = (text) => {
// Check if the input parameter is a string.
if (typeof text !== 'string') {
return 'It must be a string.';
}
// Define a regular expression pattern to match strings containing only lowercase letters.
const pattern = /^[a-z]*$/;
// Test if the input text matches the defined pattern.
return pattern.test(text);
};
// Test the function with various inputs and log the results.
console.log(test('j')); // true
console.log(test('java exercises')); // false
console.log(test('JavaScriptExercises')); // false
console.log(test('javascriptexercises')); // true
console.log(test(12356)); // 'It must be a string.'
Output:
true false false true It must be a string.
Explanation:
In the exercise above,
- The function "test()" takes one parameter, 'text', representing the input text to be tested.
- It begins with a check to ensure that the input parameter is a string. If it's not a string, the function returns the message "It must be a string."
- The function then defines a regular expression pattern (/^[a-z]*$/) that matches strings containing only lowercase letters.
- It applies this pattern using the "test()" method on the input text and returns the result.
- Test cases are provided at the end to illustrate the function's behavior with different inputs.
Flowchart:
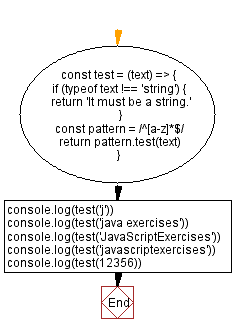
Live Demo:
See the Pen javascript-string-exercise-53 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that tests if a string is entirely lowercase with no spaces (flatcase) using regular expressions.
- Write a JavaScript function that validates the input is a string and then compares it to its lowercase version.
- Write a JavaScript function that returns true for strings that match the flatcase pattern and false otherwise.
- Write a JavaScript function that handles numeric inputs by returning an error if the input is not a string.
Improve this sample solution and post your code through Disqus.
Previous: Check exceeding word.
Next: Check a string is in Kebab case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.