JavaScript: Check exceeding word
JavaScript String: Exercise-52 with Solution
There is an increasing gap between two adjacent characters in exceeding words. In ASCII, the gap represents the distance between two characters.
Write a JavaScript program to determine if a given word is exceeding word or not.
Example:
'acgl'
Adjacent characters:
'ac' -> 2
'cg' -> 4
'gl' -> 5
So 'acgl' is exceeding word.
'aebc'
Adjacent characters:
'ae' -> 4
'eb' -> 3
'bc' -> 1
So 'aebc' is not an exceeding word.
Test Data:
'acgl' -> true
'aebc' -> false
Sample Solution:
JavaScript Code:
/**
* Source: shorturl.at/asFM4
* @function test
* @param {string} text
* @returns {boolean}
*/
const test = (word) => {
// Check if the input is a string
if (typeof word !== 'string') {
return 'It must be string';
}
// Convert the word to uppercase and remove non-alphabetic characters
const upperChars = word.toUpperCase().replace(/[^A-Z]/g, '');
// Initialize an array to store the differences between adjacent characters
const adjacentDiffList = [];
// Iterate through the characters of the word
for (let i = 0; i < upperChars.length - 1; i++) {
// Get the current character and the adjacent character
const { [i]: char, [i + 1]: adjacentChar } = upperChars;
// Calculate the difference in ASCII values between adjacent characters
if (char !== adjacentChar) {
adjacentDiffList.push(
Math.abs(char.charCodeAt() - adjacentChar.charCodeAt())
);
}
}
// Check if the adjacent differences are in non-decreasing order
for (let i = 0; i < adjacentDiffList.length - 1; i++) {
const { [i]: charDiff, [i + 1]: secondCharDiff } = adjacentDiffList;
// If the adjacent differences are not in non-decreasing order, return false
if (charDiff > secondCharDiff) {
return false;
}
}
// If all adjacent differences are in non-decreasing order, return true
return true;
};
// Test cases
console.log(test('acgl')); // Output: true
console.log(test('aebc')); // Output: false
console.log(test(12356)); // Output: "It must be string"
Output:
true false It must be a string.
Explanation:
In the exercise above,
- The function "test()" takes a single parameter 'word', which is expected to be a string.
- It first checks if the input 'word' is indeed a string. If not, it returns the message "It must be a string".
- The function then converts the input string to uppercase and removes all non-alphabetic characters using a regular expression.
- It iterates through the characters of the modified string and calculates the absolute differences in ASCII values between adjacent characters. These differences are stored in the 'adjacentDiffList' array.
- After calculating the differences, the function checks if the adjacent differences are in non-decreasing order. If any adjacent difference is greater than the next one, it returns 'false', indicating that the order is not non-decreasing.
- If all adjacent differences are in non-decreasing order, the function returns 'true', indicating that the condition is met.
Flowchart:
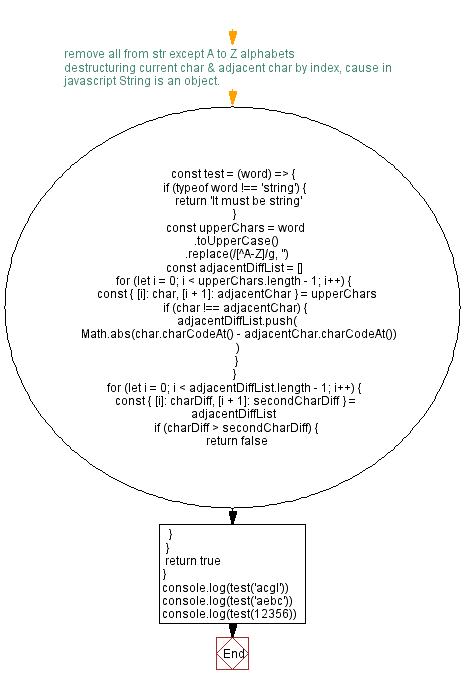
Live Demo:
See the Pen javascript-string-exercise-52 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Implement Boyer-Moore string-search algorithm.
Next: Check a string is in Flat case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-string-exercise-52.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics