JavaScript: Alphanumeric characters that are palindromes
JavaScript String: Exercise-50 with Solution
Check Alphanumeric Palindrome
A palindrome is a word, number, phrase, or other sequence of symbols that reads the same backwards as forwards, such as the words madam or racecar, the date/time stamps 11/11/11 11:11 and 02/02/2020, and the sentence: "A man, a plan, a canal – Panama". The 19-letter Finnish word saippuakivikauppias (a soapstone vendor), is the longest single-word palindrome in everyday use, while the 12-letter term tattarrattat (from James Joyce in Ulysses) is the longest in English.
Write a JavaScript program to check if a given string contains alphanumeric characters that are palindromes regardless of special characters and letter case.
Test Data:
('$22_|1372^2731|_22') -> true
('12%^&2') -> false
('234%$$%432') -> true
(1234) -> "It must be string"
('aba%$aba') -> true
('Aba%$aba') -> true
Sample Solution:
JavaScript Code:
// Define a function named test that takes an input parameter alpha_text
const test = (alpha_text) => {
// Check if the input parameter is not a string, if so, return an error message
if (typeof alpha_text !== 'string') {
return 'It must be string';
}
// Remove non-alphanumeric characters and convert the string to lowercase
const new_text = alpha_text.replace(/[^a-z0-9]+/ig, '').toLowerCase();
// Calculate the middle index of the new string
const mid_index = new_text.length >> 1;
// Iterate through the first half of the new string
for (let i = 0; i < mid_index; i++) {
// Check if the character at the current index is not equal to its counterpart from the end
if (new_text.at(i) !== new_text.at(~i)) {
// If not equal, return false (indicating that the string is not a palindrome)
return false;
}
}
// If the loop completes without finding any unequal pairs, return true (indicating that the string is a palindrome)
return true;
}
// Test the function with various inputs and print the results
console.log(test('$22_|1372^2731|_22'));
console.log(test('12%^&2'));
console.log(test('234%$$%432'));
console.log(test(1234));
console.log(test('aba%$aba'));
console.log(test('Aba%$aba'));
Output:
true false true It must be string true true
Explanation:
In the exercise above,
- The function "test()" takes one parameter 'alpha_text', representing the input string to be tested.
- It first checks if the input parameter is not a string. If it's not a string, it returns an error message 'It must be string'.
- It then removes all non-alphanumeric characters from the input string and converts it to lowercase using regular expressions and the "toLowerCase()" function.
- Next, it calculates the middle index of the cleaned string.
- It iterates through the first half of the cleaned string, comparing each character with its counterpart from the end of the string (using bitwise negation to access characters from the end).
- If any pair of characters are not equal, it returns 'false', indicating that the string is not a palindrome.
- If all pairs are equal, it returns 'true', indicating that the string is a palindrome.
- Finally, the function is tested with various inputs, and the results are printed using console.log().
Flowchart:
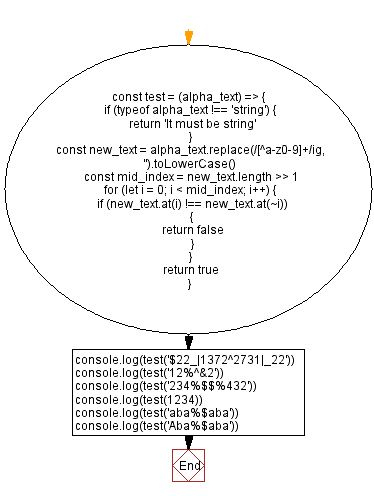
Live Demo:
See the Pen javascript-string-exercise-50 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Get unique guid of the specified length, or 32 by default.
Next: Implement Boyer-Moore string-search algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics