JavaScript: Get the successor of a string
JavaScript String: Exercise-48 with Solution
String Successor
Write a JavaScript function to get the successor to a string.
Note: The successor is calculated by incrementing characters starting from the rightmost alphanumeric (or the rightmost character if there are no alphanumerics) in the
string. Incrementing a digit always results in another digit, and incrementing a letter results in another letter of the same case. If the increment generates a carry, the character to the left of it is incremented. This process repeats until there is no carry, adding an additional character if necessary.
Example:
string.successor("abcd") == "abce"
string.successor("THX1138") == "THX1139"
string.successor("<
string.successor("1999zzz") == "2000aaa"
string.successor("ZZZ9999") == "AAAA0000"
Test Data:
console.log(successor('abcd'));
console.log(successor('3456'));
"abce"
"3457"
Sample Solution:
JavaScript Code:
// Function definition to generate the successor of a given string
function successor(str) {
// Define the alphabet and its length
var alphabet = 'abcdefghijklmnopqrstuvwxyz',
length = alphabet.length,
result = str,
i = str.length;
// Loop through each character of the string from right to left
while(i >= 0) {
// Get the last character
var last = str.charAt(--i),
next = '', // Initialize the next character
carry = false; // Flag to indicate if there's a carry operation
// Check if the last character is a letter
if (isNaN(last)) {
// Find the index of the last character in the alphabet
var index = alphabet.indexOf(last.toLowerCase());
// If the character is not in the alphabet
if (index === -1) {
next = last; // Preserve the character
carry = true; // Set carry flag
}
else {
// Determine if the last character was uppercase
var isUpperCase = last === last.toUpperCase();
// Get the next character in the alphabet
next = alphabet.charAt((index + 1) % length);
// If the original character was uppercase, make the next character uppercase
if (isUpperCase) {
next = next.toUpperCase();
}
// Check if there's a carry operation
carry = index + 1 >= length;
// If there's a carry operation and it's the first character, add a new character
if (carry && i === 0) {
var added = isUpperCase ? 'A' : 'a'; // Determine the case of the added character
result = added + next + result.slice(1); // Add the new character to the result
break;
}
}
}
else { // If the last character is a digit
next = +last + 1; // Get the next digit
// Check if there's a carry operation
if(next > 9) {
next = 0; // Reset to 0 if it's beyond 9
carry = true; // Set carry flag
}
// If there's a carry operation and it's the first character, add a new digit
if (carry && i === 0) {
result = '1' + next + result.slice(1); // Add the new digit to the result
break;
}
}
// Update the result with the next character
result = result.slice(0, i) + next + result.slice(i + 1);
// If there's no carry operation, exit the loop
if (!carry) {
break;
}
}
// Return the resulting string
return result;
}
// Test the successor function with sample input
console.log(successor('abcd'));
console.log(successor('3456'));
Output:
abce 3457
Explanation:
The above JavaScript code defines a function "successor(str)" that generates the successor of a given string. Here's a breakdown:
- The function takes a string 'str' as input.
- It initializes variables including the alphabet, the length of the alphabet, the result (initially set to the input string), and the index variable for iterating through the string from right to left.
- The function then enters a loop that iterates through each character of the string from right to left.
- Inside the loop:
- It checks if the current character is a letter or a digit.
- If it's a letter, it finds the index of the character in the alphabet and determines the next character in the alphabet. It handles uppercase characters by preserving case.
- If it's a digit, it increments it by 1, handling carry operations if necessary.
- It updates the result string with the next character or digit.
- If there's no carry operation, the loop exits.
- Finally, the function returns the resulting string.
Flowchart:
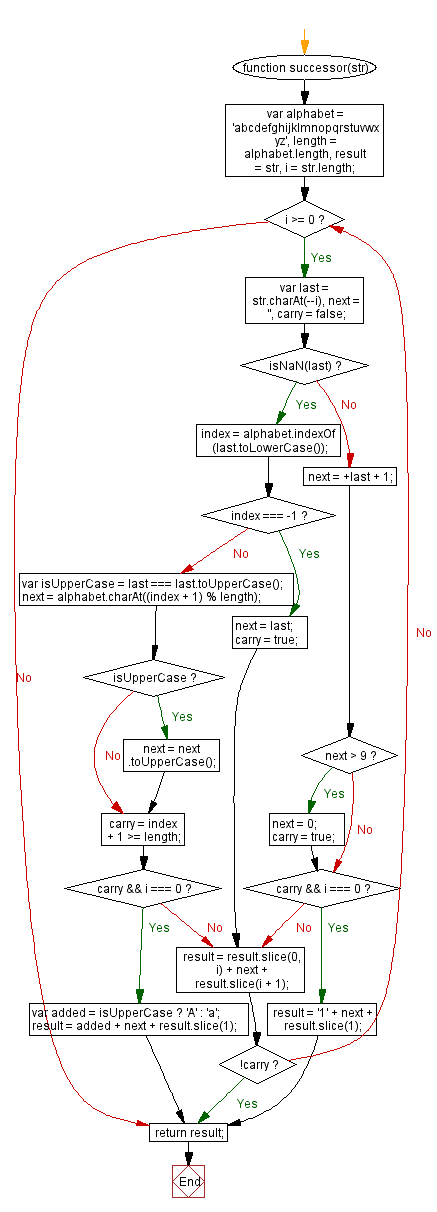
Live Demo:
See the Pen JavaScript Get the successor of a string-string-ex-48 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the successor of a string by incrementing its rightmost alphanumeric character.
- Write a JavaScript function that handles carry-over when incrementing characters in a string successor calculation.
- Write a JavaScript function that validates the input and returns the original string if no alphanumerics are found.
- Write a JavaScript function that returns the successor of a string while preserving the case of each character.
Go to:
PREV : String Successor.
NEXT : Generate GUID.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.