JavaScript: Capitalize each word in the string
JavaScript String: Exercise-41 with Solution
Capitalize Every Word
Write a JavaScript function to capitalize each word in the string.
Test Data:
console.log(capitalizeWords('js string exercises'));
"JS STRING EXERCISES"
Visual Presentation:
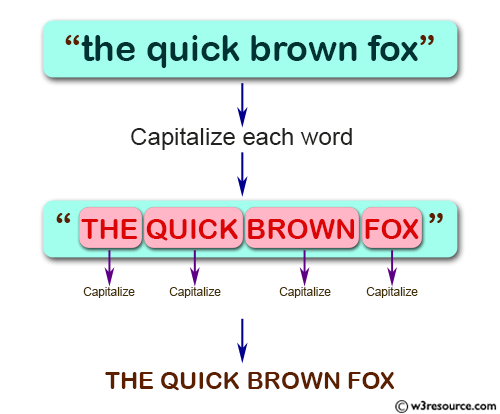
Sample Solution:
JavaScript Code:
// Define a function called capitalizeWords that takes a string str as input
function capitalizeWords(str) {
// Use the replace method with a regular expression \w\S* to match each word in the string
// For each matched word, execute a callback function
return str.replace(/\w\S*/g, function(txt) {
// Inside the callback function, convert the first character of the word to uppercase
// and keep the rest of the word unchanged
return txt.substr(0).toUpperCase();
});
}
// Call the capitalizeWords function with the input string 'js string exercises' and print the result to the console
console.log(capitalizeWords('js string exercises'));
Output:
JS STRING EXERCISES
Explanation:
In the exercise above,
- function capitalizeWords(str) {: Defines the function "capitalizeWords()" with a parameter 'str', representing the input string.
- return str.replace(/\w\S*/g, function(txt) {: Uses the "replace()" method with a regular expression '\w\S*' to match each word in the string. For each matched word, it executes a callback function.
- return txt.substr(0).toUpperCase(); });: In the callback function, it converts the first character of each word to uppercase using the "toUpperCase()" method and keeps the rest of the word unchanged.
- console.log(capitalizeWords('js string exercises'));: Calls the "capitalizeWords()" function with the input string 'js string exercises' and prints the capitalized string to the console.
Flowchart:
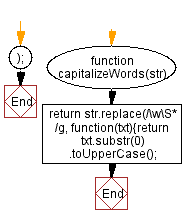
Live Demo:
See the Pen JavaScript Capitalize each word in the string-string-ex-41 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts every word in a string to uppercase.
- Write a JavaScript function that splits a string into words, converts each word entirely to uppercase, and rejoins them.
- Write a JavaScript function that uses a loop to iterate through each word and applies toUpperCase().
- Write a JavaScript function that handles punctuation by preserving non-alphabetic characters in the output.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to Uncapitalize the first letter of each word of a string.
Next: Write a JavaScript function to uncapitalize each word in the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.