JavaScript: Extract a specific number of characters from a string
JavaScript String: Exercise-4 with Solution
Extract Characters
Write a JavaScript function to extract a specified number of characters from a string.
Visual Presentation:
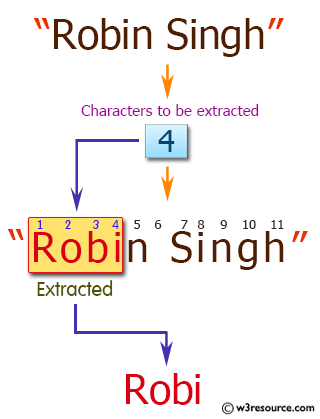
Test Data :
console.log(truncate_string("Robin Singh",4));
"Robi"
Sample Solution:
JavaScript Code:
// Define a function named truncate_string that takes two parameters: str1, representing the input string, and length, representing the maximum length of the truncated string
truncate_string = function (str1, length) {
// Check if str1 is a string and length is a positive number
if ((str1.constructor === String) && (length>0)) {
// If both conditions are met, return a substring of str1 starting from index 0 and ending at length - 1
return str1.slice(0, length);
}
};
// Call the truncate_string function with the input string "Robin Singh" and length 4, and log the result to the console
console.log(truncate_string("Robin Singh",4));
Output:
Robi
Explanation:
In the exercise above,
- The code first checks if 'str1' is a string (str1.constructor === String) and if 'length' is a positive number ('length > 0').
- If both conditions are met, it returns a substring of 'str1' starting from index 0 and ending at 'length - 1'.
- If any of the conditions are not met, the function returns 'undefined'. Finally, the code calls the "truncate_string()" function with the input string "Robin Singh" and length 4, and logs the result to the console.
Flowchart:
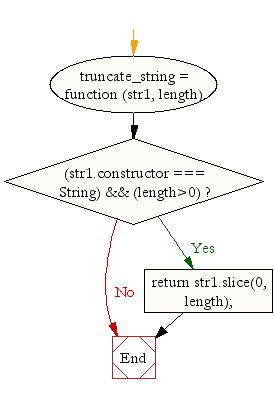
Live Demo:
See the Pen JavaScript Extract a specific number of characters from a string - string-ex-4 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that extracts the first n characters from a given string.
- Write a JavaScript function that retrieves a substring of specified length from any given position in a string.
- Write a JavaScript function that validates the length parameter and returns an empty string if n is negative.
- Write a JavaScript function that extracts characters using slice() and handles edge cases gracefully.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to split a string and convert it into an array of words.
Next: Write a JavaScript function to convert a string in abbreviated form.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.