JavaScript: Test case insensitive (except special Unicode characters) string comparison
JavaScript String: Exercise-37 with Solution
Write a JavaScript function to test case-insensitive (except special Unicode characters) string comparison.
Test Data:
console.log(compare_strings('abcd', 'AbcD'));
true
console.log(compare_strings('ABCD', 'Abce'));
false
Visual Presentation:
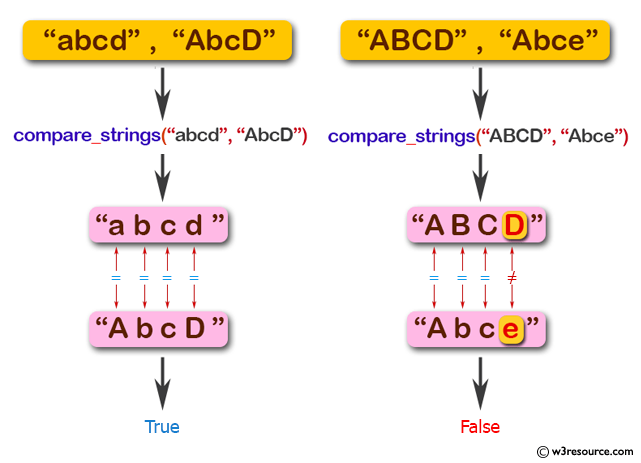
Sample Solution:
JavaScript Code:
// Define a function named compare_strings that takes two string parameters: str1 and str2
function compare_strings(str1, str2) {
// Convert both strings to uppercase and check if they are equal
var areEqual = str1.toUpperCase() === str2.toUpperCase();
// Return the result of the comparison
return areEqual;
}
// Test the function by comparing two pairs of strings and print the results
console.log(compare_strings('abcd', 'AbcD')); // Output: true
console.log(compare_strings('ABCD', 'Abce')); // Output: false
Output:
true false
Explanation:
In the exercise above,
JavaScript code defines a function called "compare_strings()" that takes two string parameters 'str1' and 'str2'. Inside the function:
- Both input strings 'str1' and 'str2' are converted to uppercase using the "toUpperCase()" method.
- The equality of the two uppercase strings is checked using the '===' operator, and the result is stored in the variable 'areEqual'.
- Finally, the function returns the boolean value 'areEqual', which indicates whether the two input strings are equal, regardless of their case.
Flowchart:
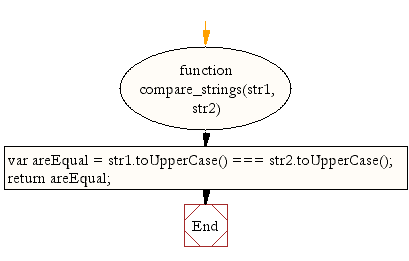
Live Demo:
See the Pen JavaScript Test case insensitive (except special Unicode characters) string comparison-string-ex-37 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to create a Zerofilled value with optional +, - sign.
Next: Write a JavaScript function to create a case-insensitive search.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-string-exercise-37.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics