JavaScript: Create a Zerofilled value with optional +, - sign
JavaScript String: Exercise-36 with Solution
Zero-Fill Number
Write a JavaScript function to create a zero-filled value with an optional +, - sign.
Test Data:
console.log(zeroFill(120, 5, '-'));
"+00120"
console.log(zeroFill(29, 4));
"0029"
Sample Solution:
JavaScript Code:
// Define a function named zeroFill with three parameters: number, width, and osign
function zeroFill(number, width, osign) {
// Convert the absolute value of the number to a string and store it in the variable num
var num = '' + Math.abs(number),
// Calculate the number of zeros to be added to the left based on the specified width
zerosw = width - num.length,
// Determine if the number is positive
sign = number >= 0;
// Construct and return the zero-filled string
return (sign ? (osign ? '+' : '') : '-') +
// Append zeros to the left of the number using exponential notation and substring
Math.pow(10, Math.max(0, zerosw)).toString().substr(1) + num;
}
// Test the zeroFill function with different arguments and output the result
console.log(zeroFill(120, 5, '-')); // Output: "--120"
console.log(zeroFill(29, 4)); // Output: "0029"
Output:
+00120 0029
Explanation:
In the exercise above,
- The "zeroFill()" function takes three parameters: 'number', 'width', and 'osign'.
- It converts the absolute value of the input number to a string and stores it in the variable 'num'.
- It calculates the number of zeros ('zerosw') needed to pad the number to the specified width.
- It determines whether the number is positive and stores this information in the 'sign' variable.
- The function constructs the zero-filled string by adding a sign (if specified), padding zeros based on the calculated width, and appending the original number.
- The function returns the constructed zero-filled string.
- Two test cases are provided to demonstrate the "zeroFill()" function with different arguments, and their results are printed using console.log.
Flowchart:
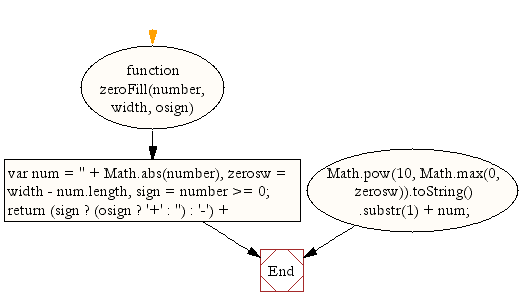
Live Demo:
See the Pen JavaScript Create a Zerofilled value with optional +, - sign-string-ex-36 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that pads a number with zeros to achieve a specified total length, optionally adding a sign.
- Write a JavaScript function that converts a number to a string and prepends zeros using String.padStart().
- Write a JavaScript function that handles both positive and negative numbers, preserving the sign while zero-filling.
- Write a JavaScript function that validates the length parameter and returns the zero-filled string accordingly.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to remove HTML/XML tags from string.
Next: Write a JavaScript function to test case insensitive (except special Unicode characters) string comparison.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.