JavaScript: Remove HTML/XML tags from string
JavaScript String: Exercise-35 with Solution
Write a JavaScript function to remove HTML/XML tags from a string.
Test Data:
console.log(strip_html_tags('<p><strong><em>PHP Exercises</em></strong></p>'));
"PHP Exercises"
Visual Presentation:
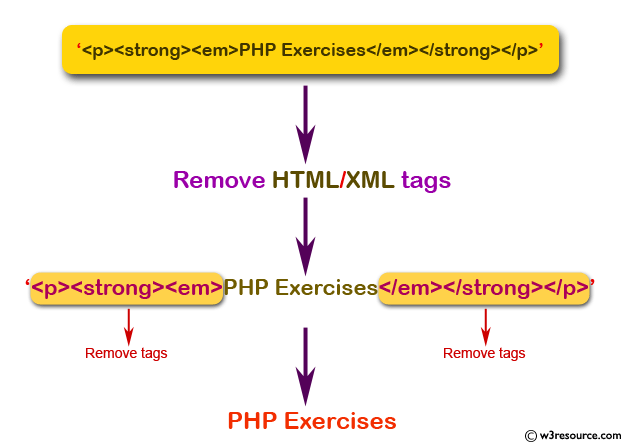
Sample Solution:
JavaScript Code:
// Function to strip HTML tags from a given string
function strip_html_tags(str) {
// Check if the input string is null or empty
if ((str === null) || (str === '')) {
// If so, return false
return false;
} else {
// If not, convert the input string to a string type
str = str.toString();
}
// Use a regular expression to replace all HTML tags with an empty string
return str.replace(/<[^>]*>/g, '');
}
// Test the function with a sample string and log the result to the console
console.log(strip_html_tags('PHP Exercises'));
Output:
PHP Exercises
Explanation:
In the exercise above,
- The function "strip_html_tags()" takes a single parameter 'str', which represents the input string from which HTML tags will be removed.
- Inside the function:
- It first checks if the input string is null or empty. If so, it returns 'false'.
- If the input string is not null or empty, it converts it to a string type.
- It then uses a regular expression '(/<[^>]*>/g)' to match and replace all HTML tags (<...>) with an empty string, effectively removing them.
- Finally, the function returns the modified string with HTML tags removed.
Flowchart:
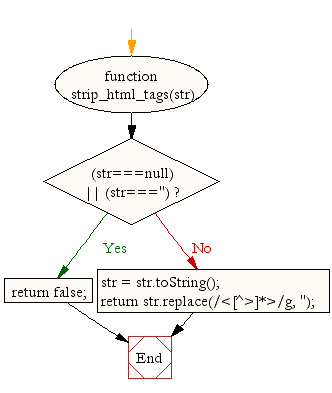
Live Demo:
See the Pen JavaScript Remove HTML/XML tags from string-string-ex-35 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert a string to title case.
Next: Write a JavaScript function to create a Zerofilled value with optional +, - sign.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-string-exercise-35.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics