JavaScript: Convert a string to title case
JavaScript String: Exercise-34 with Solution
Convert to Title Case
Write a JavaScript function to convert a string to title case.
Test Data:
console.log(sentenceCase('PHP exercises. python exercises.'));
"Php Exercises. Python Exercises."
Visual Presentation:
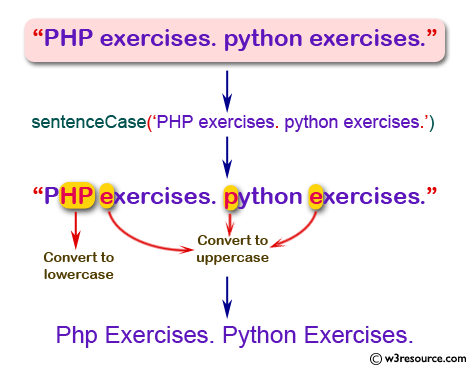
Sample Solution:
JavaScript Code:
// Define a function named sentenceCase that takes a string str as input
function sentenceCase (str) {
// Check if the input string is null or empty
if ((str===null) || (str===''))
return false;
else
// Convert the input string to a string type
str = str.toString();
// Replace each word in the string with its first letter capitalized and the rest in lowercase
return str.replace(/\w\S*/g, function(txt){return txt.charAt(0).toUpperCase() + txt.substr(1).toLowerCase();});
}
// Call the sentenceCase function with a test string and log the result to the console
console.log(sentenceCase('PHP exercises. python exercises.'));
Output:
Php Exercises. Python Exercises.
Explanation:
In the exercise above,
- The function "sentenceCase()" checks if the input string is null or empty. If so, it returns 'false'.
- If the input string is not null or empty, it is converted to a string type.
- The "replace()" method is used with a regular expression '\w\S*' to match each word in the string.
- For each match found, a callback function is invoked. Inside this function, the first character of the word is capitalized using "charAt(0).toUpperCase()", and the rest of the word is converted to lowercase using "substr(1).toLowerCase()".
- The modified words are then concatenated to form the final sentence.
- The function returns the modified sentence.
Flowchart:
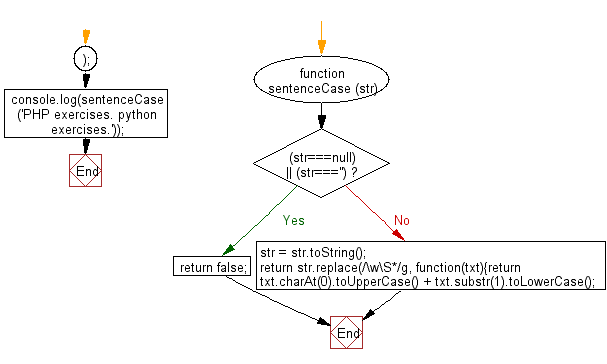
Live Demo:
See the Pen JavaScript Convert a string to title case-string-ex-34 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a string so that the first letter of each word is capitalized.
- Write a JavaScript function that splits a string into words, capitalizes each, and rejoins them with spaces.
- Write a JavaScript function that uses regular expressions to find word boundaries and apply capitalization.
- Write a JavaScript function that handles punctuation and returns the string in proper title case.
Go to:
PREV : Remove Non-Word Characters.
NEXT : Remove HTML/XML Tags.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.