JavaScript: Remove non-printable ASCII chars
JavaScript String: Exercise-32 with Solution
Remove Non-Printable ASCII
Write a JavaScript function to remove non-printable ASCII characters.
Test Data:
console.log(remove_non_ascii('äÄçÇéÉêPHP-MySQLöÖÐþúÚ'));
"PHP-MySQL"
Sample Solution:
JavaScript Code:
// Define a function named remove_non_ascii that takes a string parameter str
function remove_non_ascii(str) {
// Check if the input string is null or empty. If true, return false.
if ((str===null) || (str===''))
return false;
// Convert the input string to a string if it's not already
else
str = str.toString();
// Use the replace method with a regular expression to remove non-ASCII characters
// The regular expression /[^\x20-\x7E]/g matches any character that is not in the range of ASCII printable characters (hexadecimal values from 0x20 to 0x7E)
return str.replace(/[^\x20-\x7E]/g, '');
}
// Call the remove_non_ascii function with a test string and log the result to the console
console.log(remove_non_ascii('äÄçÇéÉêPHP-MySQLöÖÐþúÚ'));
Output:
PHP-MySQL
Explanation:
The above JavaScript code defines a function called "remove_non_ascii()" that removes non-ASCII characters from a given string. Here's a brief explanation of each part of the code:
- 'function remove_non_ascii(str) {': This line defines the function 'remove_non_ascii' that takes a string parameter 'str'.
- 'if ((str===null) || (str==='')) return false;': This line checks if the input string is null or empty. If it is, the function returns 'false'.
- 'else str = str.toString();': If the input string is not null or empty, it converts the input string to a string type if it's not already.
- 'return str.replace(/[^\x20-\x7E]/g, '');': This line uses the 'replace' method with a regular expression to remove non-ASCII characters from the string. The regular expression '/[^\x20-\x7E]/g' matches any character that is not in the range of ASCII printable characters (hexadecimal values from 0x20 to 0x7E).
- 'console.log(remove_non_ascii('äÄçÇéÉêPHP-MySQLöÖÐþúÚ'));': This line calls the "remove_non_ascii()" function with a test string ''äÄçÇéÉêPHP-MySQLöÖÐþúÚ'' and logs the result to the console. The function removes non-ASCII characters from the input string and returns the modified string.
Flowchart:
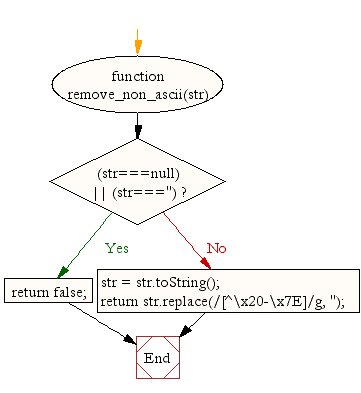
Live Demo:
See the Pen JavaScript Remove non-printable ASCII chars - string-ex-32 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that removes characters with ASCII codes below 32 from a string.
- Write a JavaScript function that uses regular expressions to filter out non-printable ASCII characters.
- Write a JavaScript function that validates the input and returns a clean string with only printable characters.
- Write a JavaScript function that processes a string and strips out control characters while preserving visible ones.
Go to:
PREV : Escape HTML Characters.
NEXT : Remove Non-Word Characters.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.