JavaScript: Escapes special characters for use in HTML
JavaScript String: Exercise-31 with Solution
Escape HTML Characters
Write a JavaScript function to escape special characters (&, <, >, ', ") for use in HTML.
Test Data:
console.log(escape_html('PHP & MySQL'));
"PHP & MySQL"
console.log(escape_html('3 > 2'));
"3 > 2"
Sample Solution:
JavaScript Code:
function escape_html(str) {
if ((str===null) || (str===''))
return false;
else
str = str.toString();
var map = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
"'": '''
};
return str.replace(/[&<>"']/g, function(m) { return map[m]; });
}
console.log(escape_html('PHP & MySQL'));
console.log(escape_html('3 > 2'));
Output:
PHP & MySQL 3 > 2
Explanation:
In the exercise above,
- The function "escape_html()" is defined to escape HTML special characters in a given string.
- It checks if the input string is null or empty. If it is, the function returns false.
- If the input string is not null or empty, it converts it to a string.
- The function defines a map object that contains HTML special characters as keys and their corresponding HTML entities as values.
- It uses a regular expression to replace occurrences of HTML special characters in the string with their corresponding HTML entities using the "replace()" method.
- Finally, the function returns the modified string with HTML special characters escaped.
Flowchart:
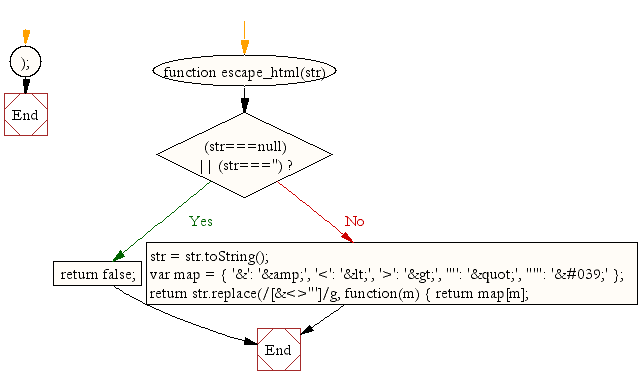
Live Demo:
See the Pen JavaScript Escapes special characters for use in HTML - string-ex-31 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that replaces &, <, >, ', and " in a string with their corresponding HTML entities.
- Write a JavaScript function that uses a map of special characters to their HTML escape sequences and applies it to a string.
- Write a JavaScript function that validates the input string and escapes only the necessary characters.
- Write a JavaScript function that iterates through a string and replaces each special character with its HTML code.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function check if a string ends with specified suffix.
Next: Write a JavaScript function to remove non-printable ASCII chars.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.