JavaScript: Check if a string ends with specified suffix
JavaScript String: Exercise-30 with Solution
String Ends With
Write a JavaScript function that checks whether a string ends with a specified suffix.
Test Data :
console.log(string_endsWith('JS PHP PYTHON','PYTHON'));
true
console.log(string_endsWith('JS PHP PYTHON',''));
false
Visual Presentation:
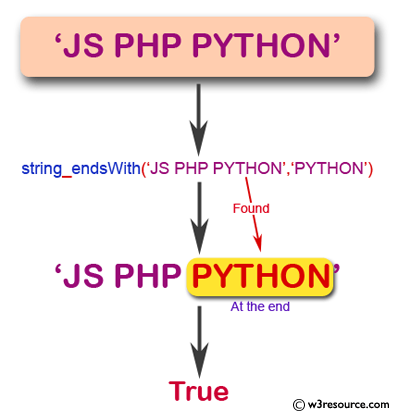
Sample Solution:
JavaScript Code:
// Function to check if a string ends with a specified suffix
function string_endsWith(str, suffix)
{
// Check if either string is null or empty
if (((str === null) || (str === '')) || ((suffix === null) || (suffix === '')))
{
return false; // Return false if either string is null or empty
}
else
{
// Convert both strings to type string
str = str.toString();
suffix = suffix.toString();
}
// Check if the suffix is found at the end of the string
return str.indexOf(suffix, str.length - suffix.length) !== -1;
}
// Example usage of the string_endsWith function
console.log(string_endsWith('JS PHP PYTHON', 'PYTHON')); // Output: true
console.log(string_endsWith('JS PHP PYTHON', '')); // Output: false
Output:
true false
Explanation:
In the exercise above,
- The function "string_endsWith()" is defined, which takes two parameters: `str` (the main string) and 'suffix' (the string to check for at the end).
- It first checks if either 'str' or 'suffix' is null or empty. If so, it returns 'false'.
- If both 'str' and 'suffix' are non-empty strings, they are converted to strings if they're not already strings.
- The function then checks if the 'suffix' is found at the end of the 'str' using the 'indexOf' method.
- If the 'suffix' is found at the end of the 'str', the function returns 'true'; otherwise, it returns 'false'.
Flowchart:
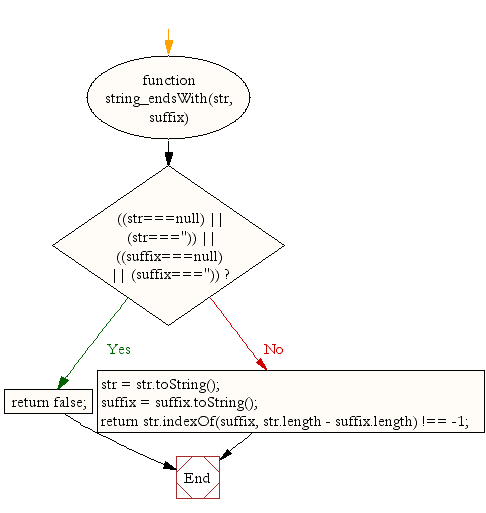
Live Demo:
See the Pen JavaScript Check if a string ends with specified suffix - string-ex-30 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a string ends with a given substring using slice() comparisons.
- Write a JavaScript function that uses the built-in endsWith() method if available, with a fallback implementation.
- Write a JavaScript function that validates the input and returns true if the string ends with the target substring.
- Write a JavaScript function that handles edge cases when the search string is empty or longer than the input.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find a word within a string.
Next: Write a JavaScript function to escapes special characters (&, <, >, ', ") for use in HTML.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.