JavaScript: Split a string and convert it into an array of words
JavaScript String: Exercise-3 with Solution
Write a JavaScript function to split a string and convert it into an array of words.
Test Data :
console.log(string_to_array("Robin Singh"));
["Robin", "Singh"]
Visual Presentation:
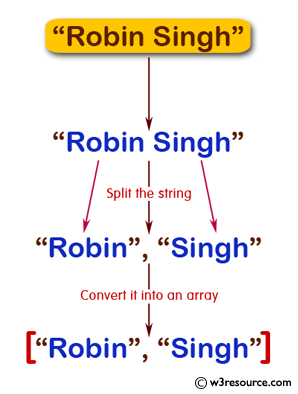
Sample Solution:
JavaScript Code:
// Define a function named string_to_array that takes a string as input
string_to_array = function (str) {
// Trim the whitespace from the beginning and end of the string, then split the string into an array using whitespace as the separator
return str.trim().split(" ");
};
// Call the string_to_array function with the input "Robin Singh" and print the result
console.log(string_to_array("Robin Singh"));
Output:
["Robin","Singh"]
Explanation:
In the exercise above,
The code defines a function called "string_to_array()" that takes a string as input. Inside the function:
- trim() is used to remove any leading or trailing whitespace from the input string.
- split(" ") is used to split the trimmed string into an array of substrings, with each substring separated by whitespace.
Flowchart:
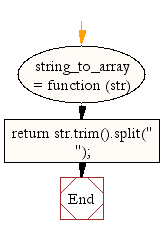
Live Demo:
See the Pen JavaScript Split a string and convert it into an array of words - string-ex-3 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to check whether a string is blank or not.
Next: Write a JavaScript function to extract a specified number of characters from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-string-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics