JavaScript: Find a word within a string
JavaScript String: Exercise-29 with Solution
Write a JavaScript function to find a word within a string.
Test Data:
console.log(search_word('The quick brown fox', 'fox'));
console.log(search_word('aa, bb, cc, dd, aa', 'aa'));
Output:
"'fox' was found 1 times."
"'aa' was found 2 times."
Visual Presentation:
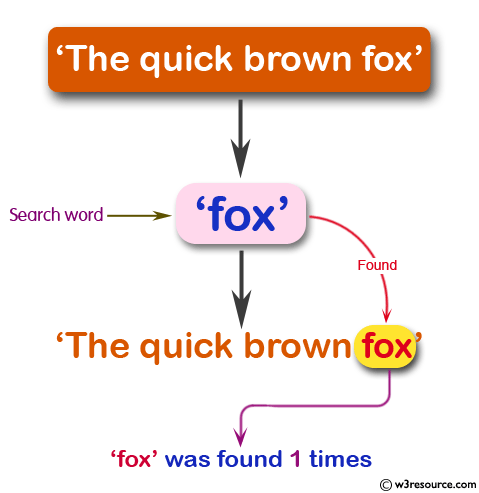
Sample Solution:
JavaScript Code:
// Define a function named search_word taking two parameters: text (the text to search within) and word (the word to search for)
function search_word(text, word){
// Initialize variables x and y to track occurrences of the word
var x = 0, y=0;
// Loop through the characters of the text string
for (i=0;i< text.length;i++)
{
// Check if the current character matches the first character of the word
if(text[i] == word[0])
{
// If a potential match is found, iterate through the characters of the word
for(j=i;j< i+word.length;j++)
{
// Check if the characters of the text match the characters of the word
if(text[j]==word[j-i])
{
// Increment y if a match is found
y++;
}
// If all characters of the word match consecutively, increment x
if (y==word.length){
x++;
}
}
// Reset y for the next potential match
y=0;
}
}
// Return the result indicating how many times the word was found in the text
return "'"+word+"' was found "+x+" times.";
}
// Demonstrate the usage of the search_word function with two example inputs and log the results to the console
console.log(search_word('The quick brown fox', 'fox'));
console.log(search_word('aa, bb, cc, dd, aa', 'aa'));
Output:
'fox' was found 1 times. 'aa' was found 2 times.
Explanation:
In the exercise above,
- The function "search_word()" takes two parameters: 'text' (the text to search within) and 'word' (the word to search for).
- It initializes two variables 'x' and 'y' to track occurrences of the word.
- It loops through the characters of the 'text' string.
- If the current character matches the first character of the 'word', it enters a nested loop to check if the consecutive characters of the 'text' match the characters of the 'word'.
- If all characters of the 'word' match consecutively, it increments x.
- It returns a string indicating how many times the 'word' was found in the 'text'.
- Finally, it demonstrates the "search_word()" function with two example inputs and logs the results to the console.
Flowchart:
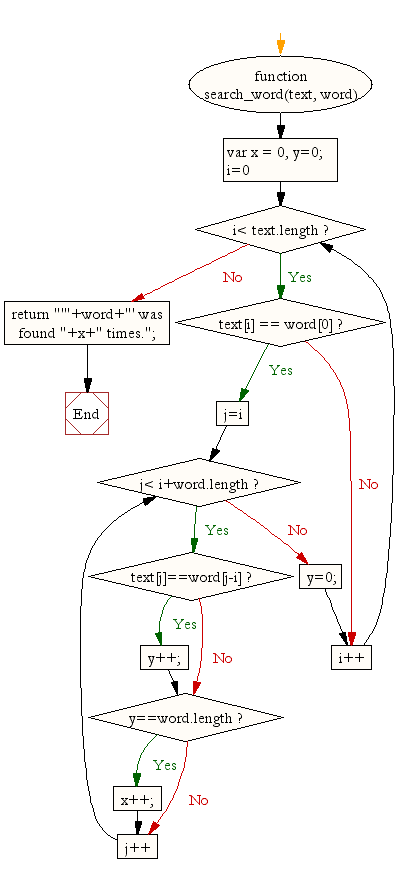
Live Demo:
See the Pen JavaScript Find a word within a string - string-ex-29 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert Hexadecimal to ASCII format.
Next: Write a JavaScript function check if a string ends with specified suffix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-string-exercise-29.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics