JavaScript: Convert Hexadecimal to ASCII format
JavaScript String : Exercise-28 with Solution
Hexadecimal to ASCII
Write a JavaScript function to convert Hexadecimal to ASCII format.
Test Data:
console.log(hex_to_ascii('3132'));
console.log(hex_to_ascii('313030'));
Output:
"12"
"100"
Sample Solution:
JavaScript Code:
// Function to convert a hexadecimal string to its ASCII representation
function hex_to_ascii(str1) {
// Convert the input hexadecimal string to a regular string
var hex = str1.toString();
// Initialize an empty string to store the resulting ASCII characters
var str = '';
// Iterate through the hexadecimal string, processing two characters at a time
for (var n = 0; n < hex.length; n += 2) {
// Extract two characters from the hexadecimal string and convert them to their ASCII equivalent
str += String.fromCharCode(parseInt(hex.substr(n, 2), 16));
}
// Return the resulting ASCII string
return str;
}
// Example usage of the hex_to_ascii function
console.log(hex_to_ascii('3132')); // Output: '12'
console.log(hex_to_ascii('313030')); // Output: '100'
Output:
12 100
Explanation:
In the exercise above,
The "hex_to_ascii()" function takes a hexadecimal string 'str1' as input and converts it into its corresponding ASCII representation. Here's a breakdown of each part:
- var hex = str1.toString();: Convert the input hexadecimal string to a regular string and store it in the variable 'hex'.
- var str = '';: Initialize an empty string 'str' which stores the resulting ASCII characters.
- for (var n = 0; n < hex.length; n += 2) {: Iterate through the hexadecimal string in steps of 2 characters.
- str += String.fromCharCode(parseInt(hex.substr(n, 2), 16));: Extract two characters from the hexadecimal string starting at index n, convert them to their decimal equivalent using 'parseInt', and then convert the decimal value to its corresponding ASCII character using 'String.fromCharCode()'. Append this ASCII character to the 'str' string.
- return str;: Return the resulting ASCII string.
Flowchart:
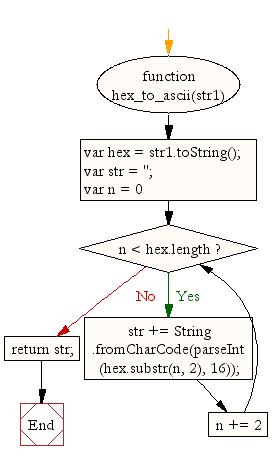
Live Demo:
See the Pen JavaScript Convert Hexadecimal to ASCII format - string-ex-28 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a hexadecimal string to its ASCII text by processing each pair of hex digits.
- Write a JavaScript function that iterates through a hex string and decodes it into characters.
- Write a JavaScript function that validates the hex input and returns an error if invalid characters are present.
- Write a JavaScript function that supports both uppercase and lowercase hex digits during conversion.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert ASCII to Hexadecimal format.
Next: Write a JavaScript function to find a word within a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics