JavaScript: Remove the first occurrence of a given 'search string' from a string
JavaScript String: Exercise-26 with Solution
Remove First Occurrence
Write a JavaScript function to remove the first occurrence of a given 'search string' from a string.
Test Data:
console.log(remove_first_occurrence("The quick brown fox jumps over the lazy dog", 'the'));
Output:
"The quick brown fox jumps over lazy dog"
Visual Presentation:
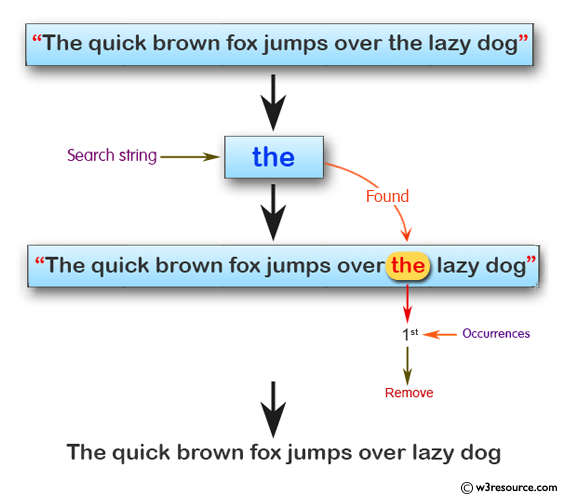
Sample Solution:
JavaScript Code:
// Define a function called remove_first_occurrence which takes two parameters: str (the main string) and searchstr (the substring to be removed)
function remove_first_occurrence(str, searchstr) {
// Find the index of the first occurrence of searchstr within str
var index = str.indexOf(searchstr);
// If searchstr is not found in str, return str as it is
if (index === -1) {
return str;
}
// If searchstr is found in str, return a new string that concatenates the substring before the first occurrence of searchstr with the substring after searchstr
return str.slice(0, index) + str.slice(index + searchstr.length);
}
// Call the remove_first_occurrence function with a sample input and log the result to the console
console.log(remove_first_occurrence("The quick brown fox jumps over the lazy dog", 'the'));
Output:
The quick brown fox jumps over lazy dog
Explanation:
The above JavaScript code defines a function called "remove_first_occurrence()" that takes two parameters: 'str' (the main string) and 'searchstr' (the substring to be removed).
- The function finds the index of the first occurrence of 'searchstr' within 'str'.
- If 'searchstr' is not found in 'str', the function returns 'str' as it is.
- If 'searchstr' is found in 'str', the function returns a new string that concatenates the substring before the first occurrence of 'searchstr' with the substring after 'searchstr'.
Flowchart:
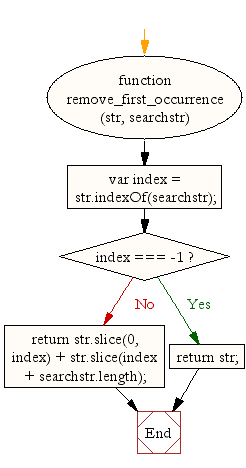
Live Demo:
See the Pen Remove the first occurrence of a given 'search string' from a string - string-ex-26 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that removes the first occurrence of a given substring from a string.
- Write a JavaScript function that uses indexOf() and slice() to eliminate the first match of a target substring.
- Write a JavaScript function that handles cases where the search string is not found by returning the original string.
- Write a JavaScript function that validates inputs and returns a modified string with the first occurrence removed.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to alphabetize a given string.
Next: Write a JavaScript function to convert ASCII to Hexadecimal format.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.