JavaScript: Get a part of string after a specified character
JavaScript String: Exercise-22 with Solution
Substring After Character
Write a JavaScript function to get a part of string after a specified character.
Test Data:
console.log(subStrAfterChars('w3resource: JavaScript Exercises', ':','a'));
console.log(subStrAfterChars('w3resource: JavaScript Exercises', 'E','b'));
Output:
"w3resource"
"xercises"
Sample Solution:
JavaScript Code:
// Define a function named subStrAfterChars with parameters str (input string), char (character to search for), and pos (position indicator).
function subStrAfterChars(str, char, pos)
{
// If the position indicator is 'b' (before), return the substring after the specified character.
if(pos=='b')
return str.substring(str.indexOf(char) + 1);
// If the position indicator is 'a' (after), return the substring before the specified character.
else if(pos=='a')
return str.substring(0, str.indexOf(char));
// If the position indicator is neither 'a' nor 'b', return the original string.
else
return str;
}
// Test the subStrAfterChars function by printing the substring after ':' with the position indicator 'a'.
console.log(subStrAfterChars('w3resource: JavaScript Exercises', ':','a'));
// Test the subStrAfterChars function by printing the substring before 'E' with the position indicator 'b'.
console.log(subStrAfterChars('w3resource: JavaScript Exercises', 'E','b'));
Output:
w3resource xercises
Explanation:
In the exercise above,
- The "subStrAfterChars()" function takes three parameters: 'str' (the input string), 'char' (the character to search for), and 'pos' (the position indicator).
- Inside the function, it checks the value of the 'pos 'parameter:
- If 'pos' is 'b' (before), it returns the substring after the specified character using 'substring' and 'indexOf' functions.
- If 'pos' is 'a' (after), it returns the substring before the specified character.
- If 'pos' is neither 'a' nor 'b', it returns the original string.
- The function is tested with two examples using console.log, demonstrating how to extract substrings before and after specific characters.
Flowchart:
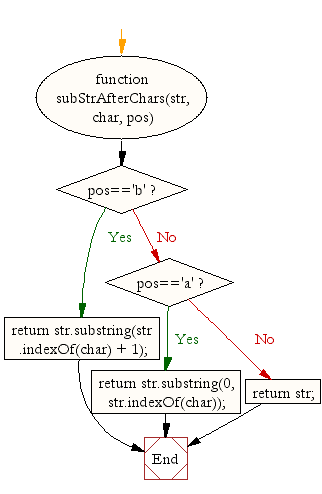
Live Demo:
See the Pen JavaScript Get a part of string after a specified character - string-ex-22 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the part of a string after the first occurrence of a specified character.
- Write a JavaScript function that handles cases where the character does not exist by returning the original string.
- Write a JavaScript function that accepts a string and a delimiter, and splits the string at the delimiter to return the latter portion.
- Write a JavaScript function that validates the delimiter input and returns a custom message if not found.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to repeat a string a specified times.
Next: Write a JavaScript function to strip leading and trailing spaces from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.