JavaScript: Pad (left, right) a string to get to a determined length
JavaScript String: Exercise-20 with Solution
Write a JavaScript function that can pad (left, right) a string to get to a specific length.
Test Data:
console.log(formatted_string('0000',123,'l'));
console.log(formatted_string('00000000',123,''));
Output:
"0123"
"12300000"
Visual Presentation:
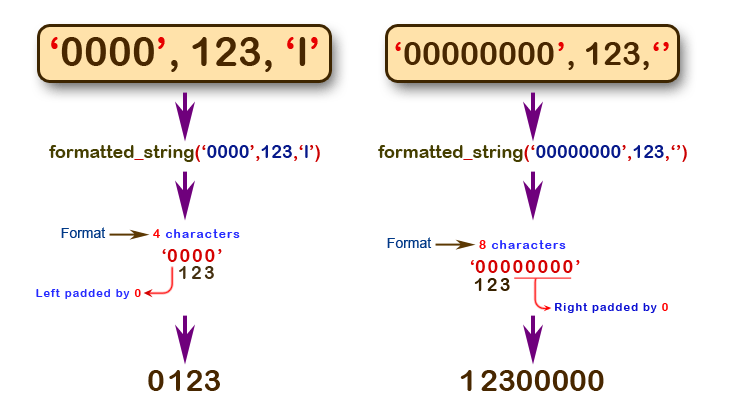
Sample Solution:
JavaScript Code:
// Define a function named formatted_string with three parameters: pad, user_str, and pad_pos
function formatted_string(pad, user_str, pad_pos) {
// Check if user_str is undefined, if so, return pad
if (typeof user_str === 'undefined')
return pad;
// Check if pad_pos is 'l' (left), if true, execute the following block
if (pad_pos == 'l') {
// Concatenate pad with user_str, slice the result to keep only the last characters equal to the length of pad, and return the result
return (pad + user_str).slice(-pad.length);
}
// If pad_pos is not 'l', execute the following block
else {
// Concatenate user_str with pad, then substring to keep only the characters equal to the length of pad, and return the result
return (user_str + pad).substring(0, pad.length);
}
}
// Test the function with different parameters and log the results to the console
console.log(formatted_string('0000',123,'l'));
console.log(formatted_string('00000000',123,''));
Output:
0123 12300000
Explanation:
The above JavaScript code defines a function "formatted_string()" that takes three parameters: 'pad', 'user_str', and 'pad_pos'. Here's a brief explanation -
- The function checks if 'user_str' is 'undefined'. If so, it returns 'pad'.
- If 'pad_pos' is 'l' (indicating left padding), it concatenates 'pad' with 'user_str', keeps only the last characters equal to the length of 'pad', and returns the result.
- If 'pad_pos' is not 'l', it concatenates 'user_str' with 'pad', keeps only the characters equal to the length of 'pad', and returns the result.
Flowchart:
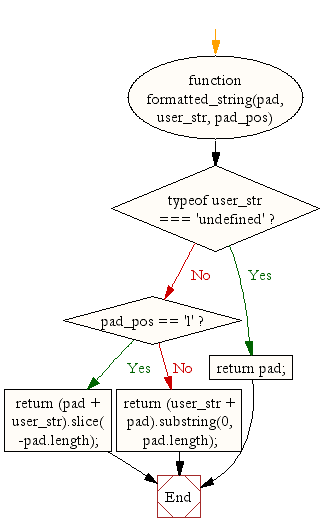
Live Demo:
See the Pen JavaScript Pad (left, right) a string to get to a determined length - string-ex-20 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Reverse a Binary string.
Next: Repeat a string a specified times.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-string-exercise-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics