JavaScript: Check whether a string is blank or not
JavaScript String: Exercise-2 with Solution
Check Blank String
Write a JavaScript function to check whether a string is blank or not.
Test Data :
console.log(is_Blank(''));
console.log(is_Blank('abc'));
true
false
Visual Presentation:
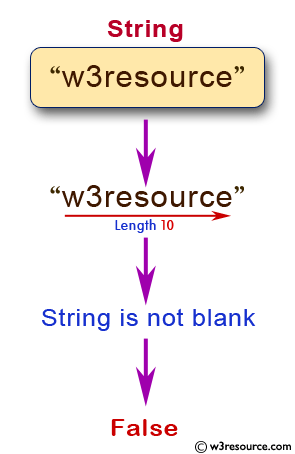
Sample Solution:
JavaScript Code:
// Define a function called is_Blank that checks if the input string is blank
is_Blank = function(input) {
// Check if the length of the input string is 0
if (input.length === 0)
// If the length is 0, return true indicating that the string is blank
return true;
else
// If the length is not 0, return false indicating that the string is not blank
return false;
}
// Print the result of calling is_Blank with an empty string
console.log(is_Blank(''));
// Print the result of calling is_Blank with a non-empty string ('abc')
console.log(is_Blank('abc'));
Output:
true false
Explanation:
In the exercise above,
- "is_Blank()" function is defined without input validation.
- Inside the function, it checks if the length of the input string is equal to 0, indicating that the string is empty.
- If the length is 0, it returns 'true', indicating that the string is blank.
- If the length is not 0, it returns 'false', indicating that the string is not blank.
- The code then tests the function by calling it with an empty string '' and a non-empty string 'abc'.
- It prints the result of each function call using console.log.
Flowchart:
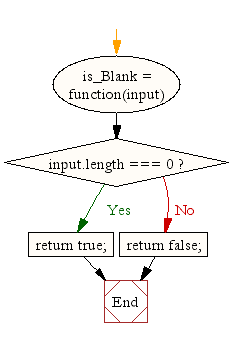
Live Demo:
See the Pen JavaScript Check whether a string is blank or not - string-ex-2 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a string is empty or contains only whitespace using trim().
- Write a JavaScript function that returns true if a string is blank and false if it contains any non-space characters.
- Write a JavaScript function that uses regular expressions to test for blank strings.
- Write a JavaScript function that validates a string input and returns a boolean indicating its blankness.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to check whether an 'input' is a string or not.
Next: Write a JavaScript function to split a string and convert it into an array of words.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.