JavaScript: Reverse a Binary string
JavaScript String: Exercise-19 with Solution
Write a JavaScript function that takes a positive integer and reverses the binary representation of that integer. Finally return the decimal version of the binary string.
Test Data:
(100) -> 19
Explanation:
Binary representation of 100 is 1100100
Reverse of 1100100 is 10011
Decimal form of 10011 is 19
(1134) -> 945
Explanation:
Binary representation of 1134 is 10001101110
Reverse of 10001101110 is 1110110001
Decimal form of 1110110001 is 945
Sample Solution:
JavaScript Code:
function test(n) {
return parseInt(n.toString(2).split('').reverse().join(''), 2);
}
console.log(test(100));
console.log(test(1134));
Output:
19 945
Explanation:
In the exercise above,
- The function takes an integer 'n' as input.
- It converts the integer n to its binary representation using the "toString(2)" method, which returns a string containing the binary representation of the number.
- It splits the binary string into an array of characters, reverses the order of the characters, and then joins them back into a single string using the "reverse()" and "join('')" methods.
- Finally, it converts the reversed binary string back to an integer using "parseInt(string, 2)", where the second argument specifies that the string is in base 2 (binary).
- The function returns a reversed binary integer.
- The function is tested with different input numbers, and the results are printed to the console.
Flowchart:
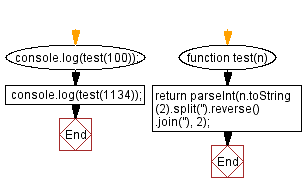
Live Demo:
See the Pen javascript-string-exercise-64 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Count the occurrence of a substring in a string.
Next: Pad (left, right) a string to get to a determined length
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-string-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics