JavaScript: Count the occurrence of a substring in a string
JavaScript String: Exercise-18 with Solution
Count Substring Occurrences
Write a JavaScript function to count substrings in a string.
Test Data:
console.log(count("The quick brown fox jumps over the lazy dog", 'the'));
Output:
2
console.log(count("The quick brown fox jumps over the lazy dog", 'fox',false));
Output:
1
Visual Presentation:
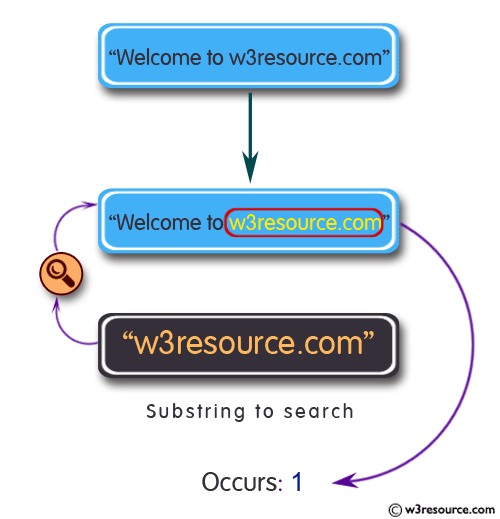
Sample Solution-1:
JavaScript Code:
// Define a function named count that takes two parameters: main_str (the main string) and sub_str (the substring to count)
function count(main_str, sub_str)
{
// Convert main_str and sub_str to strings if they are not already
main_str += '';
sub_str += '';
// If sub_str is an empty string or undefined, return the length of main_str plus 1
if (sub_str.length <= 0)
{
return main_str.length + 1;
}
// Escape special characters in sub_str for use in regular expression
subStr = sub_str.replace(/[.*+?^${}()|[\]\\]/g, '\\$&');
// Count the occurrences of sub_str in main_str using a regular expression and return the count
return (main_str.match(new RegExp(subStr, 'gi')) || []).length;
}
// Test the count function with sample input strings and log the results to the console
console.log(count("The quick brown fox jumps over the lazy dog", 'the'));
console.log(count("The quick brown fox jumps over the lazy dog", 'fox',false));
console.log(count("The quick brown fox jumps over the lazy dog", 'fox',false));
Output:
2 1
Explanation:
In the exercise above,
- The "count()" function takes two parameters: 'main_str' (the main string) and 'sub_str' (the substring to count).
- It converts both 'main_str' and 'sub_str' to strings if they are not already strings.
- If 'sub_str' is an empty string or undefined, the function returns the length of 'main_str' plus 1.
- It escapes special characters in 'sub_str' using a regular expression.
- It counts the occurrences of 'sub_str' in 'main_str' using a global case-insensitive regular expression match and returns the count.
- The function is tested with sample input strings, and the results are logged to the console.
Flowchart:
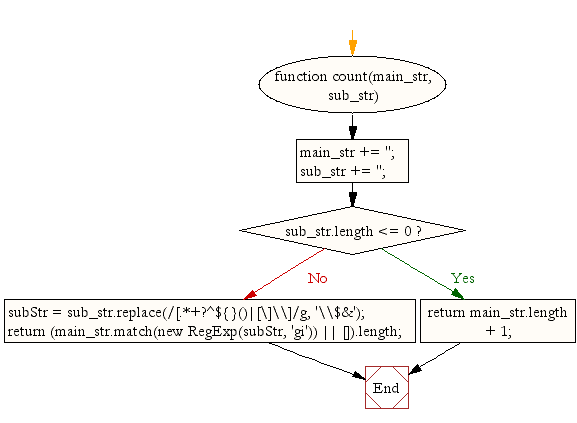
Sample Solution-2:
Counts the occurrences of a substring in a given string.
- Use Array.prototype.indexOf() to look for searchValue in str.
- Increment a counter if the value is found and update the index, i.
- Use a while loop that will return as soon as the value returned from Array.prototype.indexOf() is -1.
JavaScript Code:
// Source: https://bit.ly/3hEZdCl
// Function to count the occurrences of a substring within a main string
const count_substr = (str, searchValue) => {
let count = 0, // Initialize a counter variable
i = 0; // Initialize an index variable
// Loop until the end of the string is reached
while (true) {
// Find the index of the next occurrence of the searchValue in the string starting from index i
const r = str.indexOf(searchValue, i);
// If the searchValue is found in the string
if (r !== -1) {
// Increment the counter by 1 and update the index to start searching from the next position
[count, i] = [count + 1, r + 1];
} else {
// If the searchValue is not found, return the final count
return count;
}
}
};
// Test cases
console.log(count_substr("The quick brown fox jumps over the lazy dog", 'the'));
console.log(count_substr("The quick brown fox jumps over the lazy dog", 'fox'));
Output:
1 1
Explanation:
The above JavaScript code defines a function "count_substr()" that counts the occurrences of a substring within a main string. It iterates through the main string using 'indexOf' to find each occurrence of the substring, updating a counter variable accordingly. Finally, it returns the total count of occurrences.
Finally two test cases demonstrate its usage by counting the occurrences of different substrings within given main strings.
Flowchart:
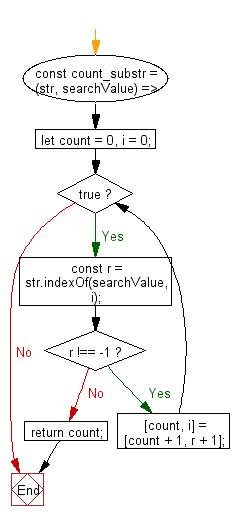
Live Demo:
See the Pen JavaScript Count the occurrence of a substring in a string - string-ex-18 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that counts the occurrences of a substring in a given string, case-sensitive by default.
- Write a JavaScript function that accepts a flag to perform a case-insensitive count of a substring.
- Write a JavaScript function that uses regular expressions to count overlapping occurrences of a substring.
- Write a JavaScript function that validates the inputs and returns an error if the substring is empty.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to chop a string into chunks of a given length.
Next: Reverse a Binary string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.