JavaScript: Chop a string into chunks of a specific length
JavaScript String: Exercise-17 with Solution
Write a JavaScript function to chop a string into chunks of a given length.
Test Data:
console.log(string_chop('w3resource'));
console.log(string_chop('w3resource',2));
console.log(string_chop('w3resource',3));
["w3resource"]
["w3", "re", "so", "ur", "ce"]
["w3r", "eso", "urc", "e"]
Visual Presentation:
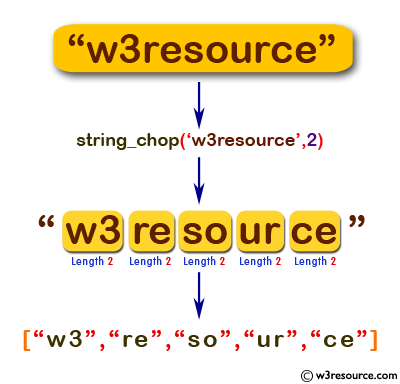
Sample Solution:-
JavaScript Code:
// Define a function called string_chop, taking two parameters: str (the input string) and size (the size of each chunk).
string_chop = function(str, size){
// Check if the input string is null; if so, return an empty array.
if (str == null) return [];
// Convert the input string to a string if it's not already.
str = String(str);
// Convert the size parameter to an integer using the bitwise NOT operator.
size = ~~size;
// Check if the size is greater than 0; if so, split the string into chunks of the specified size using a regular expression and return the result as an array.
return size > 0 ? str.match(new RegExp('.{1,' + size + '}', 'g')) : [str];
}
// Test the function with different input strings and sizes, and log the results to the console.
console.log(string_chop('w3resource'));
console.log(string_chop('w3resource',2));
console.log(string_chop('w3resource',3));
Output:
["w3resource"] ["w3","re","so","ur","ce"] ["w3r","eso","urc","e"]
Explanation:
In the exercise above,
- The function "string_chop()" takes two parameters: 'str' (the input string) and 'size' (the size of each chunk).
- It checks if the input string is 'null'. If it is, it returns an empty array.
- It converts the input string to a string if it doesn't already have one.
- It converts the size parameter to an integer using the bitwise NOT operator.
- If the size is greater than 0, it splits the string into chunks of the specified size using a regular expression (.{1,size}) and returns the result as an array. If the size is less than or equal to 0, it returns an array containing the original string.
- The code then tests the function with different input strings and sizes and logs the results to the console.
Flowchart:
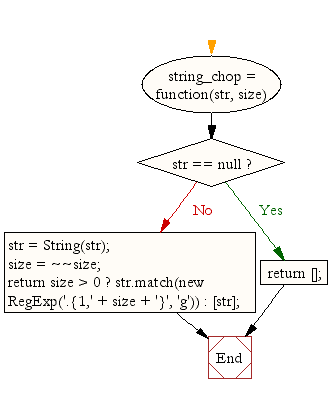
Live Demo:
See the Pen JavaScript Chop a string into chunks of a specific length - string-ex-17 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to truncate a string if it is longer than the specified number of characters.
Next: Write a JavaScript function to count the occurrence of a substring in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics