JavaScript: Truncate a string if it is longer than the specified number of characters
JavaScript String: Exercise-16 with Solution
Truncate String with Ellipsis
Write a JavaScript function to truncate a string if it is longer than the specified number of characters. Truncated strings will end with a translatable ellipsis sequence ("…") (by default) or specified characters.
Test Data:
console.log(text_truncate('We are doing JS string exercises.'))
console.log(text_truncate('We are doing JS string exercises.',19))
console.log(text_truncate('We are doing JS string exercises.',15,'!!'))
"We are doing JS string exercises."
"We are doing JS ..."
"We are doing !!"
Sample Solution:
JavaScript Code:
// Define a function named text_truncate that takes three parameters: str (the input string), length (the maximum length of the output string), and ending (the optional ending to append if the string is truncated)
text_truncate = function(str, length, ending) {
// If the length parameter is not provided, set it to 100 characters
if (length == null) {
length = 100;
}
// If the ending parameter is not provided, set it to '...'
if (ending == null) {
ending = '...';
}
// Check if the length of the input string exceeds the specified length
if (str.length > length) {
// If yes, truncate the string to length - ending.length characters and append the ending
return str.substring(0, length - ending.length) + ending;
} else {
// If no, return the original string
return str;
}
};
// Test the function with different input strings and output the results to the console
console.log(text_truncate('We are doing JS string exercises.'))
console.log(text_truncate('We are doing JS string exercises.',19))
console.log(text_truncate('We are doing JS string exercises.',15,'!!'))
Output:
We are doing JS string exercises. We are doing JS ... We are doing !!
Explanation:
In the exercise above,
- The function "text_truncate()" takes three parameters: 'str' (the input string), 'length' (the maximum length of the output string), and 'ending' (an optional ending to append if the string is truncated).
- Inside the function:
- If the 'length' parameter is not provided, it defaults to 100 characters.
- If the 'ending' parameter is not provided, it defaults to '...'.
- It checks if the length of the input string ('str.length') exceeds the specified maximum length ('length').
- If the input string is longer than the maximum length, it truncates the string to 'length - ending.length' characters and appends the 'ending' string.
- If the input string is not longer than the maximum length, it returns the original input string.
- Write a JavaScript function that truncates a string to a specified length and appends an ellipsis (or custom ending) if truncated.
- Write a JavaScript function that validates the maximum length and returns the original string if it is shorter.
- Write a JavaScript function that allows an optional custom truncation suffix instead of the default ellipsis.
- Write a JavaScript function that handles edge cases like zero or negative truncation length.
Flowchart:
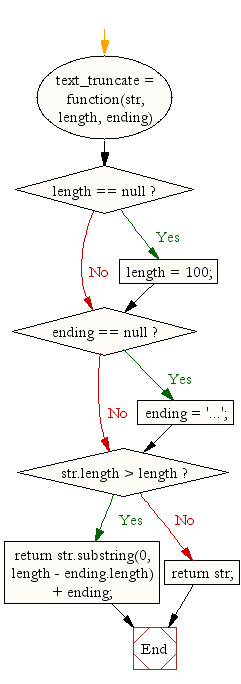
Live Demo:
See the Pen JavaScript Truncate a string if it is longer than the specified number of characters - string-ex-16 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to humanized number (Formats a number to a human-readable string.) with the correct suffix such as 1st, 2nd, 3rd or 4th.
Next: Write a JavaScript function to chop a string into chunks of a given length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics