JavaScript: Formats a number to a human-readable string
JavaScript String: Exercise-15 with Solution
Humanize Format
Write a JavaScript function that format a number in a human-readable string with the correct suffix, such as 1st, 2nd, 3rd, etc.
Test Data:
console.log(humanize_format());
console.log(humanize_format(1));
console.log(humanize_format(8));
console.log(humanize_format(301));
console.log(humanize_format(402));
"1st"
"8th"
"301st"
"402nd"
Sample Solution:
JavaScript Code:
// Define a function named humanize_format that takes a number num as input
humanize_format = function humanize(num) {
// Check if the num parameter is undefined, return undefined if so
if(typeof(num) == "undefined") return;
// Check if the number modulo 100 falls within the range 11-13, return the number followed by "th" if true
if(num % 100 >= 11 && num % 100 <= 13)
return num + "th";
// Switch statement to handle different cases based on the last digit of the number
switch(num % 10) {
// Return the number followed by "st" if the last digit is 1
case 1: return num + "st";
// Return the number followed by "nd" if the last digit is 2
case 2: return num + "nd";
// Return the number followed by "rd" if the last digit is 3
case 3: return num + "rd";
}
// Return the number followed by "th" for all other cases
return num + "th";
}
// Output the result of calling the humanize_format function with different test cases
console.log(humanize_format());
console.log(humanize_format(1));
console.log(humanize_format(8));
console.log(humanize_format(301));
console.log(humanize_format(402));
Output:
undefined 1st 8th 301st 402nd
Explanation:
In the exercise above,
- The function "humanize_format()" takes a number as input and returns a human-readable format for ordinal numbers.
- It first checks if the input number is undefined and returns undefined if so.
- It then checks if the number modulo 100 falls within the range 11-13. If true, it returns the number followed by "th".
- If the number is not in the special case range, it uses a switch statement to handle different cases based on the last digit of the number:
- If the last digit is 1, it returns the number followed by "st".
- If the last digit is 2, it returns the number followed by "nd".
- If the last digit is 3, it returns the number followed by "rd".
- For all other cases, it returns the number followed by "th".
- The code then outputs the results of calling the "humanize_format()" function with different test cases to the console.
Flowchart:
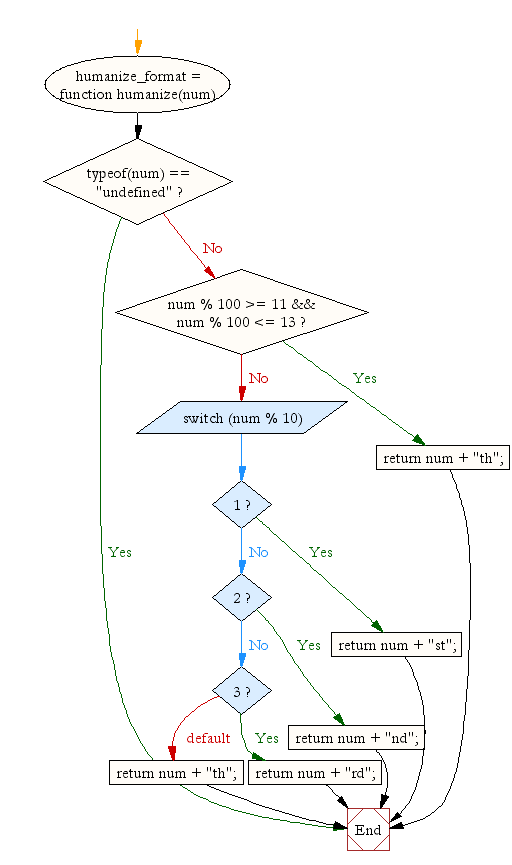
Live Demo:
See the Pen JavaScript Formats a number to a human-readable string - string-ex-15 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a number to a humanized string with the correct ordinal suffix (e.g., 1st, 2nd, 3rd).
- Write a JavaScript function that checks for special cases like 11, 12, and 13 when determining the ordinal suffix.
- Write a JavaScript function that formats numbers with suffixes and handles zero and negative values appropriately.
- Write a JavaScript function that takes an input number and returns the humanized string, ensuring proper type validation.
Go to:
PREV : Insert in String.
NEXT : Truncate String with Ellipsis.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.