JavaScript: Insert a string within a specific position in another string
JavaScript String: Exercise-14 with Solution
Insert in String
Write a JavaScript function to insert a string within a string at a particular position (default is 1).
Test Data:
console.log(insert('We are doing some exercises.'));
console.log(insert('We are doing some exercises.','JavaScript '));
console.log(insert('We are doing some exercises.','JavaScript ',18));
"We are doing some exercises."
"JavaScript We are doing some exercises."
"We are doing some JavaScript exercises."
Visual Presentation:
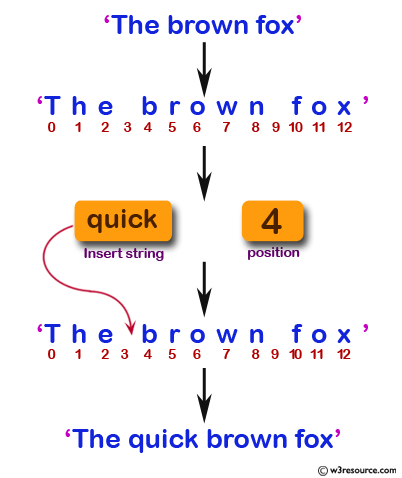
Sample Solution:
JavaScript Code:
// Define a function named insert that takes three parameters:
// main_string (the main string where ins_string will be inserted)
// ins_string (the string to insert into main_string)
// pos (the position in main_string where ins_string will be inserted)
insert = function insert(main_string, ins_string, pos) {
// If pos is not provided, default it to 0
if(typeof(pos) == "undefined") {
pos = 0;
}
// If ins_string is not provided, default it to an empty string
if(typeof(ins_string) == "undefined") {
ins_string = '';
}
// Insert ins_string into main_string at position pos
return main_string.slice(0, pos) + ins_string + main_string.slice(pos);
}
// Test cases to demonstrate the functionality of the insert function
console.log(insert('We are doing some exercises.'));
console.log(insert('We are doing some exercises.','JavaScript '));
console.log(insert('We are doing some exercises.','JavaScript ',18));
Output:
We are doing some exercises. JavaScript We are doing some exercises. We are doing some JavaScript exercises.
Explanation:
In the exercise above,
- The "insert()" function takes three parameters: 'main_string', 'ins_string', and 'pos'.
- If the 'pos' parameter is not provided, it defaults to 0.
- If the 'ins_string' parameter is not provided, it defaults to an empty string.
- The function then inserts the 'ins_string' into the 'main_string' at the specified position 'pos'.
- The modified 'main_string' with the 'ins_string' inserted is returned.
The provided test cases demonstrate the usage of the "insert()" function with different scenarios:
- Inserting nothing into the string "We are doing some exercises." at the beginning (position 0).
- Inserting "JavaScript " into the string "We are doing some exercises." at the beginning (position 0).
- Inserting "JavaScript " into the string "We are doing some exercises." at position 18.
Flowchart:
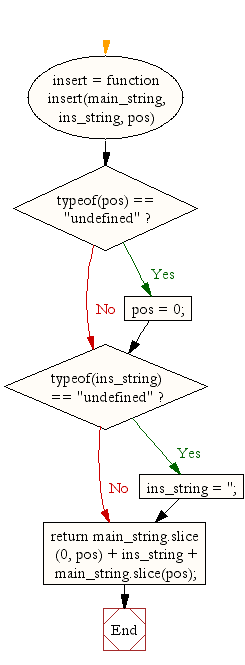
Live Demo:
See the Pen JavaScript Insert a string within a specific position in another string - string-ex-14 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that inserts a given substring into another string at a specified position.
- Write a JavaScript function that defaults to inserting at the beginning if no position is provided.
- Write a JavaScript function that validates the insertion index and handles cases where it exceeds the string length.
- Write a JavaScript function that uses slice() to split the original string and then concatenates the inserted string in the middle.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to concatenates a given string n times (default is 1).
Next: Write a JavaScript function to humanized number (Formats a number to a human-readable string.) with the correct suffix such as 1st, 2nd, 3rd or 4th.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.