JavaScript: Concatenate a specific string for a specific number of times
JavaScript String: Exercise-13 with Solution
Repeat String
Write a JavaScript function to concatenate a given string n times (default is 1).
Test Data:
console.log(repeat('Ha!'));
console.log(repeat('Ha!',2));
console.log(repeat('Ha!',3));
"Ha!"
"Ha!Ha!"
"Ha!Ha!Ha!"
Visual Presentation:
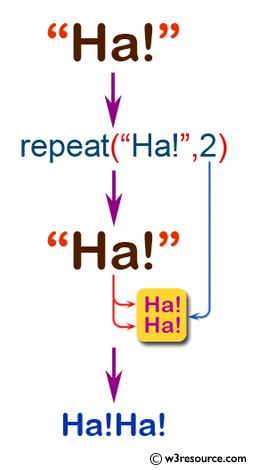
Sample Solution:
JavaScript Code:
// Define a function called repeat that takes a string str and an optional count parameter
repeat = function repeat(str, count) {
// If count parameter is not provided, set it to 1
if(typeof(count) == "undefined") {
count =1;
}
// Return an empty string if count is less than 1, otherwise repeat the string count times
return count < 1 ? '' : new Array(count + 1).join(str);
}
// Test the repeat function with different inputs and print the results to the console
console.log(repeat('Ha!'));
console.log(repeat('Ha!',2));
console.log(repeat('Ha!',3));
Output:
Ha! Ha!Ha! Ha!Ha!Ha!
Explanation:
In the exercise above,
- The "repeat()" function takes two parameters: 'str' (the string to repeat) and 'count' (the number of times to repeat the string).
- If the 'count' parameter is not provided, it defaults to 1.
- The function checks if 'count' is less than 1. If so, it returns an empty string, indicating no repetition.
- If 'count' is 1 or greater, the function creates an array with a length of 'count + 1' and then joins the elements of the array with the 'str' string to repeat it 'count' times.
- The results of calling the "repeat()" function with different inputs are printed to the console for testing.
Flowchart:
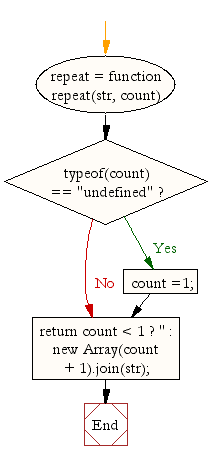
Live Demo:
See the Pen JavaScript Concatenate a specific string for a specific number of times - string-ex-13 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that repeats a string a specified number of times using a loop.
- Write a JavaScript function that employs recursion to repeat a string n times.
- Write a JavaScript function that uses the repeat() method if available, and falls back to a custom implementation otherwise.
- Write a JavaScript function that validates the count parameter and returns an error if it is not a positive integer.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to uncamelize a string.
Next: Write a JavaScript function to insert a string within a string at a particular position (default is 1).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.