JavaScript: Convert a string into camel case
JavaScript String: Exercise-11 with Solution
Camelize String
Write a JavaScript function to convert a string into camel case.
Test Data:
console.log(camelize("JavaScript Exercises"));
console.log(camelize("JavaScript exercises"));
console.log(camelize("JavaScriptExercises"));
"JavaScriptExercises"
"JavaScriptExercises"
"JavaScriptExercises"
Visual Presentation:
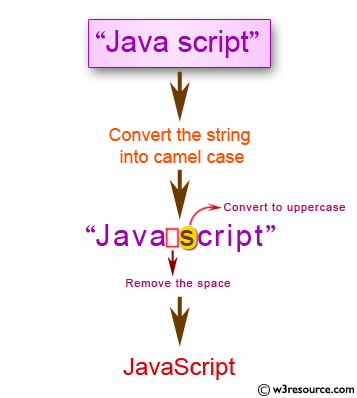
Sample Solution:
JavaScript Code:
// Define a function named camelize, taking a string parameter 'str'
camelize = function camelize(str) {
// Use the replace method with a regular expression to match non-word characters followed by any character
// The regular expression /\W+(.)/g matches one or more non-word characters followed by any character, capturing the character
// For each match, a callback function is invoked with 'match' representing the entire match and 'chr' representing the captured character
return str.replace(/\W+(.)/g, function(match, chr) {
// Convert the captured character to uppercase and return it
return chr.toUpperCase();
});
}
// Output the result of camelize function for different input strings
console.log(camelize("JavaScript Exercises"));
console.log(camelize("JavaScript exercises"));
console.log(camelize("JavaScriptExercises"));
Output:
JavaScriptExercises JavaScriptExercises JavaScriptExercises
Explanation:
In the exercise above,
- The function "camelize()" takes a string 'str' as input.
- Inside the function, the "replace()" method is used on the input string 'str'.
- The regular expression '/\W+(.)/g' is used to match non-word characters followed by any character and captures that character.
- For each match found, a callback function is executed, where 'match' represents the entire matched substring and 'chr' represents the captured character.
- Within the callback function, the captured character 'chr' is converted to uppercase using the "toUpperCase()" method.
- The "replace()" method replaces each matched substring with its corresponding uppercase character.
- Finally, the function returns the modified string in camel case format.
Flowchart:
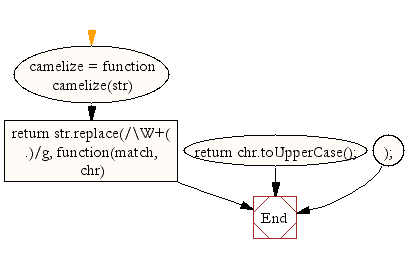
Live Demo:
See the Pen JavaScript Convert a string into camel case - string-ex-11 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a string with spaces into camelCase format.
- Write a JavaScript function that removes non-alphanumeric characters before converting a string to camelCase.
- Write a JavaScript function that handles multiple delimiters and outputs a properly camelized string.
- Write a JavaScript function that verifies if an already camelized string remains unchanged after processing.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that takes a string which has lower and upper case letters as a parameter and converts upper case letters to lower case, and lower case letters to upper case.
Next: Write a JavaScript function to uncamelize a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.