JavaScript: Input a string and converts upper case letters to lower and vice versa
JavaScript String: Exercise-10 with Solution
Swap Case
Write a JavaScript function that takes a string with both lowercase and upper case letters as a parameter. It converts upper case letters to lower case, and lower case letters to upper case.
Test Data:
console.log(swapcase('AaBbc'));
"aAbBC"
Visual Presentation:
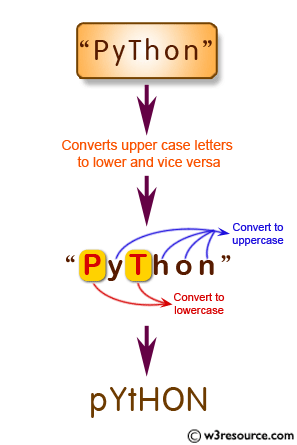
Sample Solution:
JavaScript Code:
// Define a function named swapcase that takes a string str as input
swapcase = function swapcase(str) {
// Use the replace method with a regular expression to match lowercase and uppercase letters separately
return str.replace(/([a-z]+)|([A-Z]+)/g, function(match, chr) {
// For each match, if chr (lowercase letter) exists, convert it to uppercase; otherwise, convert the match (uppercase letter) to lowercase
return chr ? match.toUpperCase() : match.toLowerCase();
});
}
// Output the result of applying the swapcase function to the string 'AaBbc'
console.log(swapcase('AaBbc'));
Output:
aAbBC
Explanation:
In the exercise above,
- The function "swapcase()" is declared, taking a string 'str' as input.
- Inside the function, a regular expression is used to match lowercase and uppercase letters separately.
- The "replace()" method is applied to the string 'str', replacing each match:
- If the match is a lowercase letter ('([a-z]+)'), it is replaced with its uppercase equivalent.
- If the match is an uppercase letter ('([A-Z]+)'), it is replaced with its lowercase equivalent.
- The modified string is returned by the function.
- Finally, the console.log statement calls the "swapcase()" function with the string 'AaBbc' as input and outputs the result.
Flowchart:
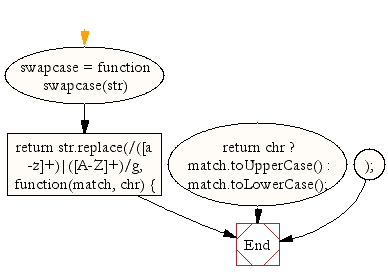
Live Demo:
See the Pen JavaScript Input a string and converts upper case letters to lower and vice versa - string-ex-10 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that iterates through a string and converts uppercase letters to lowercase and vice versa.
- Write a JavaScript function that uses split() and map() to swap the case of each character in a string.
- Write a JavaScript function that employs a for loop to build a new string with each character's case toggled.
- Write a JavaScript function that validates the input type before swapping the case and returns an error if not a string.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to capitalize the first letter of each word in a string.
Next: Write a JavaScript function to convert a string into camel case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.