JavaScript: Check whether an 'input' is a string or not
JavaScript String: Exercise-1 with Solution
Check String Input
Write a JavaScript function to check whether an 'input' is a string or not.
Test Data:
console.log(is_string('w3resource'));
true
console.log(is_string([1, 2, 4, 0]));
false
Visual Presentation:
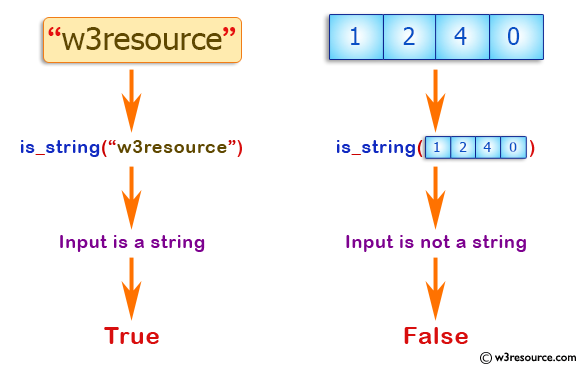
Sample Solution:
JavaScript Code:
// Define a function named is_string that takes an input parameter
is_string = function(input) {
// Check if the type of input is a string by examining its internal [[Class]] property
if (Object.prototype.toString.call(input) === '[object String]')
// Return true if the input is a string
return true;
else
// Return false if the input is not a string
return false;
};
// Output the result of calling the is_string function with a string argument
console.log(is_string('w3resource'));
// Output the result of calling the is_string function with an array argument
console.log(is_string([1, 2, 4, 0]));
Output:
true false
Explanation:
In the exercise above,
- The "is_string()" function is defined, taking one parameter as input.
- Inside the function, it checks the type of 'input' by using Object.prototype.toString.call('input').
- If the result of this check matches the string '[object String]', it returns 'true', indicating that the input is a string.
- Otherwise, it returns 'false', indicating that the input is not a string.
- The function definition ends.
- Two examples are provided to demonstrate the "is_string()" function with different types of input: a string ('w3resource') and an array ([1, 2, 4, 0]).
- The results of calling "is_string()" with these inputs are printed to the console using console.log.
Flowchart:
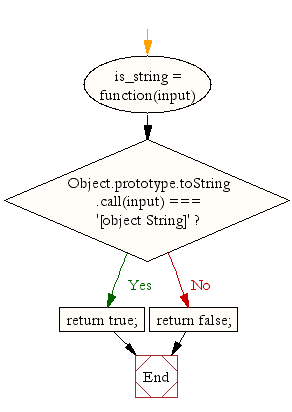
Live Demo:
See the Pen JavaScript Print an integer with commas as thousands separators - string-ex-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a variable is of type string using typeof and Object.prototype.toString.
- Write a JavaScript function that determines if an input is a string by verifying it has a substring method.
- Write a JavaScript function that accepts various inputs and returns true only for string primitives (not String objects).
- Write a JavaScript function that tests an input and returns a custom error if it is not a string.
Improve this sample solution and post your code through Disqus.
Previous: Javascript String Exercises.
Next: Write a JavaScript function to check whether a string is blank or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.