JavaScript: Compute the exponent of a number
JavaScript Function: Exercise-5 with Solution
Exponentiation
Write a JavaScript program to compute the exponent of a number.
Note: The exponent of a number says how many times the base number is used as a factor.
82 = 8 x 8 = 64. Here 8 is the base and 2 is the exponent.
Visual Presentation:
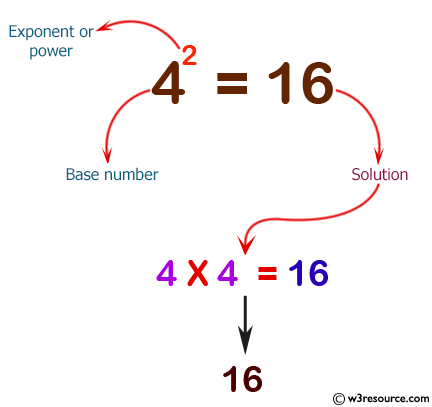
Sample Solution-1:
JavaScript Code:
// Recursive JavaScript function to calculate the exponent (a^n).
var exponent = function(a, n) {
// Base case: if n is 0, return 1.
if (n === 0) {
return 1;
} else {
// Recursive case: a^n = a * a^(n-1).
return a * exponent(a, n - 1);
}
};
// Example usage: Calculate and print the exponentiation of 4^2.
console.log(exponent(4, 2));
Output:
16
Flowchart:
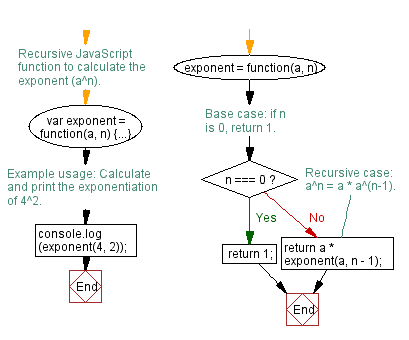
Live Demo:
See the Pen javascript-recursion-function-exercise-5 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Recursive JavaScript function to calculate the exponent (a^n).
function exponent(base, power) {
if (power === 0) {
return 1;
} else if (power % 2 === 0) {
// If the power is even, use the formula (a^(n/2))^2.
let temp = exponent(base, power / 2);
return temp * temp;
} else {
// If the power is odd, use the formula a * a^(n-1).
return base * exponent(base, power - 1);
}
}
// Example usage: Calculate and print the exponentiation of 4^2.
console.log(exponent(4, 2));
Output:
16
Flowchart:
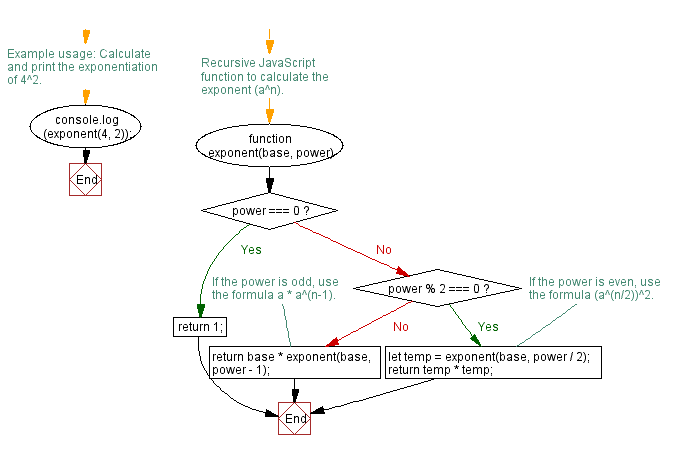
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates b^n using recursion with the exponentiation by squaring method.
- Write a JavaScript function that computes the exponent of a number iteratively without using Math.pow.
- Write a JavaScript function that recursively computes the power of a number and validates the input types.
- Write a JavaScript function that calculates b^n using recursion and handles negative exponents by computing reciprocals.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to compute the sum of an array of integers.Next: Write a JavaScript program to get the first n Fibonacci numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.