JavaScript: Get the integers in a range
JavaScript Function: Exercise-3 with Solution
Range of Integers Using Recursion
Write a JavaScript program to get integers in the range (x, y) using recursion.
Example : range(2, 9)
Expected Output : [3, 4, 5, 6, 7, 8]
Visual Presentation:
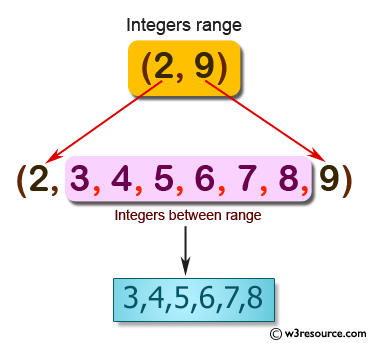
Sample Solution-1:
JavaScript Code:
// Function to generate a range of numbers between start_num and end_num (exclusive).
var range = function(start_num, end_num)
{
// Base case: if the difference between end_num and start_num is 2,
// return an array containing the number between them.
if (end_num - start_num === 2)
{
return [start_num + 1];
}
else
{
// Recursive case: generate the range between start_num and end_num - 1,
// then push the current end_num - 1 to the array.
var list = range(start_num, end_num - 1);
list.push(end_num - 1);
return list;
}
};
// Example usage: Generate and print the range of numbers between 2 and 9 (exclusive).
console.log(range(2, 9));
Output:
[3,4,5,6,7,8]
Flowchart:
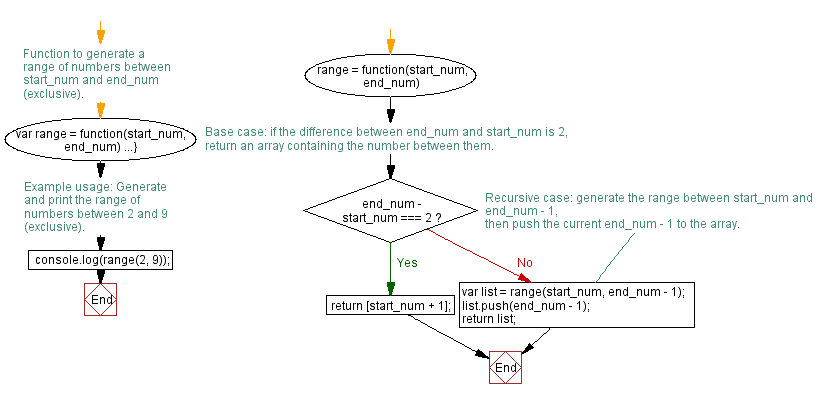
Live Demo:
See the Pen javascript-recursion-function-exercise-3 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to get integers in the range (x, y) using recursion.
function getRangeIntegers(x, y, result = []) {
// Base case: if x is equal to or greater than y, return the result.
if (x >= y - 1) {
return result;
} else {
// Recursive case: increment x and push it to the result array.
result.push(x + 1);
return getRangeIntegers(x + 1, y, result);
}
}
// Example usage: Get and print integers in the range (2, 9).
console.log(getRangeIntegers(2, 9));
Output:
[3,4,5,6,7,8]
Flowchart:
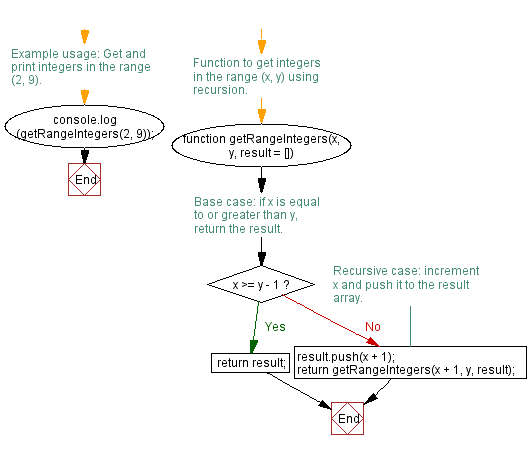
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns an array of integers between two numbers recursively, excluding the endpoints.
- Write a JavaScript function that generates a range of integers recursively, supporting both ascending and descending orders.
- Write a JavaScript function that recursively creates a range of integers and throws an error if the start is greater than the end.
- Write a JavaScript function that uses recursion to generate a range of integers and returns the result in reverse order.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to find the greatest common divisor (gcd) of two positive numbers.
Next: Write a JavaScript program to compute the sum of an array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.