JavaScript: Find the greatest common divisor of two positive numbers
JavaScript Function: Exercise-2 with Solution
GCD Using Recursion
Write a JavaScript program to find the greatest common divisor (GCD) of two positive numbers using recursion.
Visual Presentation:
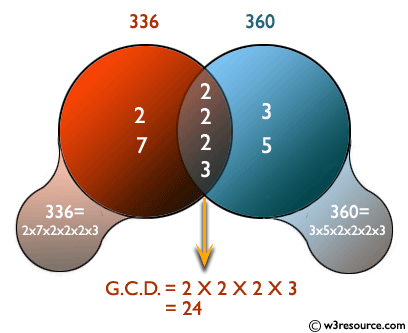
Sample Solution-1:
JavaScript Code:
// Function to calculate the greatest common divisor (GCD) of two numbers using Euclidean algorithm.
var gcd = function(a, b) {
// Base case: if b is 0, then GCD is a.
if (!b) {
return a;
}
// Recursive case: calculate GCD using the remainder (a % b).
return gcd(b, a % b);
};
// Example usage: Calculate and print the GCD of 2154 and 458.
console.log(gcd(2154, 458));
Output:
2
Flowchart:
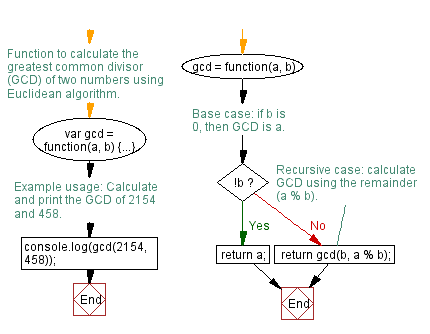
Live Demo:
See the Pen javascript-recursion-function-exercise-2 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to calculate the GCD of two numbers using recursion.
function gcdRecursive(a, b) {
// Ensure both numbers are positive.
a = Math.abs(a);
b = Math.abs(b);
// Base case: if one of the numbers is 0, the other number is the GCD.
if (b === 0) {
return a;
}
// Recursive case: calculate GCD using the remainder (a % b).
return gcdRecursive(b, a % b);
}
// Example usage: Calculate and print the GCD of 2154 and 458.
console.log(gcdRecursive(2154, 458));
Output:
2
Flowchart:
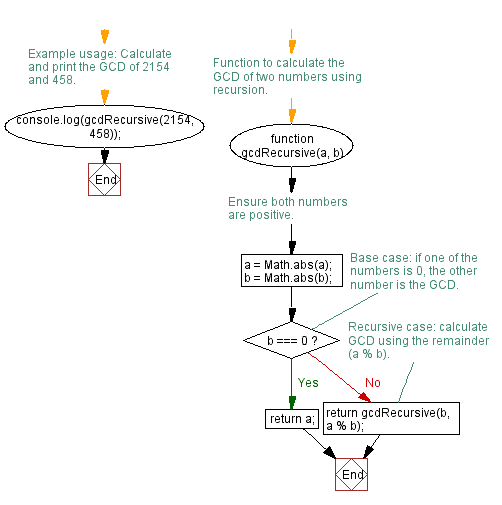
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the greatest common divisor of two numbers using the Euclidean algorithm recursively.
- Write a JavaScript function that computes the GCD recursively and handles non-integer inputs by validating them.
- Write a JavaScript function that uses recursion along with destructuring assignment to calculate the GCD of two numbers.
- Write a JavaScript function that recursively calculates the GCD and correctly handles the case when one of the numbers is zero.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to calculate the factorial of a number.
Next: Write a JavaScript program to get the integers in range (x, y).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.