JavaScript: Calculate the factorial of a number
JavaScript Function: Exercise-1 with Solution
Write a JavaScript program to calculate the factorial of a number.
In mathematics, the factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n. For example, 5! = 5 x 4 x 3 x 2 x 1 = 120
Visual Presentation:
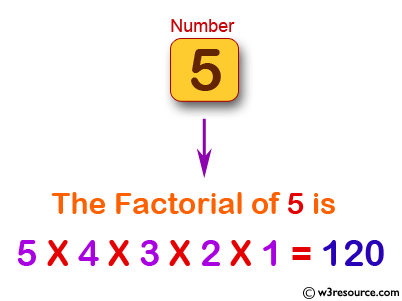
Sample Solution-1:
JavaScript Code:
// Recursive JavaScript function to calculate the factorial of a number.
function factorial(x) {
// Base case: factorial of 0 is 1.
if (x === 0) {
return 1;
}
// Recursive case: x! = x * (x-1)!
return x * factorial(x - 1);
}
// Example usage: Calculate and print the factorial of 5.
console.log(factorial(5));
Output:
120
Flowchart:
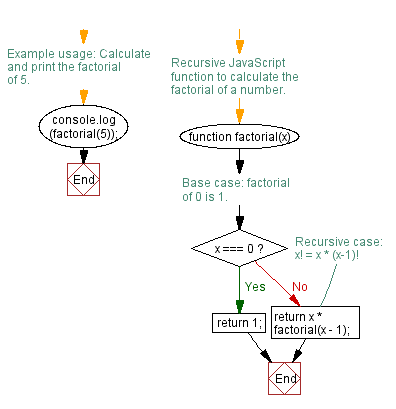
Live Demo:
See the Pen javascript-recursion-function-exercise-1 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Recursive JavaScript function to calculate the factorial of a number.
function factorial(x, result = 1) {
// Base case: factorial of 0 or 1 is 1.
if (x === 0 || x === 1) {
return result;
}
// Recursive case: x! = x * (x-1)!
return factorial(x - 1, x * result);
}
// Example usage: Calculate and print the factorial of 5.
console.log(factorial(5));
Output:
120
Flowchart:
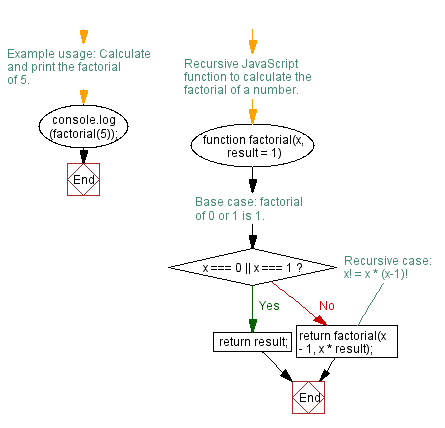
Improve this sample solution and post your code through Disqus.
Previous: Javascript Recursion Functions Exercises
Next: Write a JavaScript program to find the greatest common divisor (gcd) of two positive numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-recursion-function-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics