JavaScript: Retrieve all the names of object's own and inherited properties
JavaScript Object: Exercise-13 with Solution
Object Properties (Own & Inherited)
Write a JavaScript function to retrieve all the names of an object's own and inherited properties.
Sample Solution: -
JavaScript Code:
function allKeys(obj) {
if (!isObject(obj)) return [];
var keys = [];
for (var key in obj) keys.push(key);
return keys;
}
function isObject(obj)
{
var type = typeof obj;
return type === 'function' || type === 'object' && !!obj;
}
function Student(name) {
this.name = name;
}
Student.prototype.rollno = true;
console.log(allKeys(new Student("Sara")));
Output:
["name","rollno"]
Flowchart:
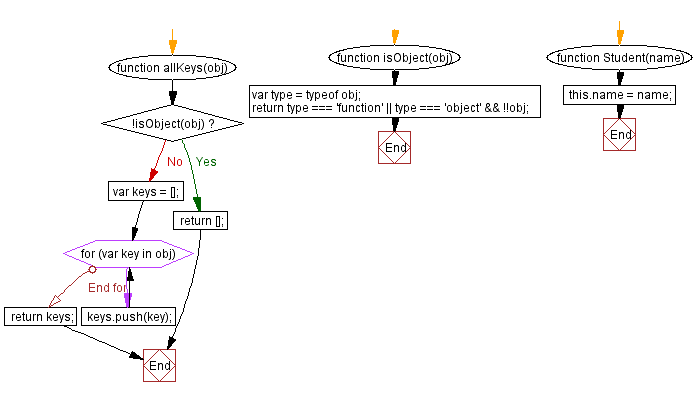
Live Demo:
See the Pen javascript-object-exercise-13 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that retrieves all own and inherited property names from an object using a for...in loop.
- Write a JavaScript function that distinguishes between an object's own properties and those inherited from its prototype.
- Write a JavaScript function that lists all property names, including non-enumerable ones, from a given object.
- Write a JavaScript function that returns an object with two arrays: one for own properties and one for inherited properties.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to parse an URL.
Next: Write a JavaScript function to retrieve all the values of an object's properties.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.