JavaScript: Column number that relates to a column title
JavaScript Math: Exercise-98 with Solution
Excel Column Number from Title
Write a JavaScript program to get the column number (integer value) related to a column title as it appears in an Excel sheet.
For example:
A -> 1
B -> 2
C -> 3
...
Z -> 26
AA -> 27
AB -> 28
...
Visual Presentation:
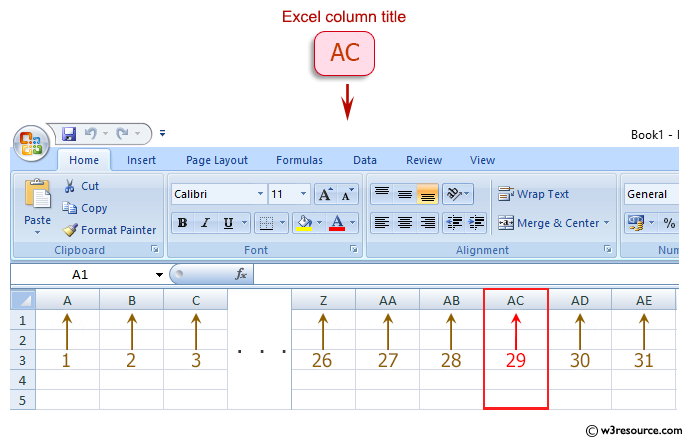
Test Data:
("C") -> 3
("AD") -> 30
("ZX") -> 700
Sample Solution-1:
JavaScript Code:
/**
* Function to convert an Excel column title to its corresponding column number.
* @param {string} text - The Excel column title.
* @returns {number} - The column number.
*/
function test(text) {
text = text.toUpperCase(); // Convert the text to uppercase
var cl_no = 0; // Initialize the column number
var len = text.length; // Get the length of the text
for (var i = 0; i < len; i++) { // Iterate through each character in the text
cl_no += (Math.pow(26, (len - i - 1)) * (text.charCodeAt(i) - 64)); // Calculate the column number
}
return cl_no; // Return the column number
}
// Test cases
text = "C";
console.log("Original text: " + text);
console.log("Excel column title related with the said column number: " + test(text));
text = "AD";
console.log("Original text: " + text);
console.log("Excel column title related with the said column number: " + test(text));
text = "ZX";
console.log("Original text: " + text);
console.log("Excel column title related with the said column number: " + test(text));
Output:
Original text: C Excel column title related with the said column number: 3 Original text: AD Excel column title related with the said column number: 30 Original text: ZX Excel column title related with the said column number: 700
Flowchart:
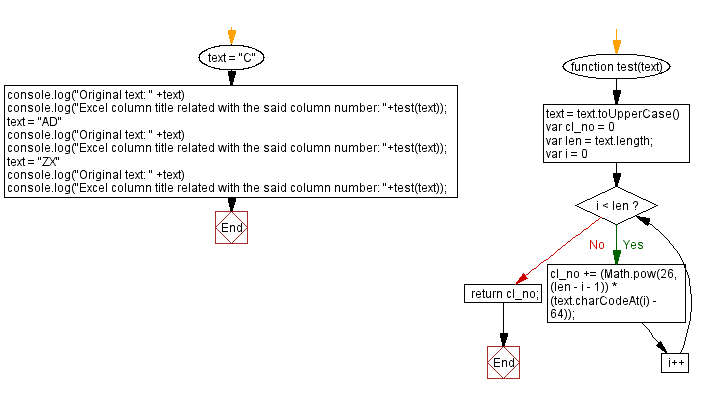
Live Demo:
See the Pen javascript-math-exercise-98 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
/**
* Function to convert an Excel column title to its corresponding column number.
* @param {string} text - The Excel column title.
* @returns {number} - The column number.
*/
function test(text) {
col_num = 0; // Initialize the column number
for (el of text) { // Loop through each character in the text
col_num = col_num * 26 + el.toLowerCase().charCodeAt(0) - "a".charCodeAt(0) + 1; // Calculate the column number
}
return col_num; // Return the column number
}
// Test cases
text = "C";
console.log("Original text: " + text);
console.log("Excel column title related with the said column number: " + test(text));
text = "AD";
console.log("Original text: " + text);
console.log("Excel column title related with the said column number: " + test(text));
text = "ZX";
console.log("Original text: " + text);
console.log("Excel column title related with the said column number: " + test(text));
Output:
Original text: C Excel column title related with the said column number: 3 Original text: AD Excel column title related with the said column number: 30 Original text: ZX Excel column title related with the said column number: 700
Flowchart:
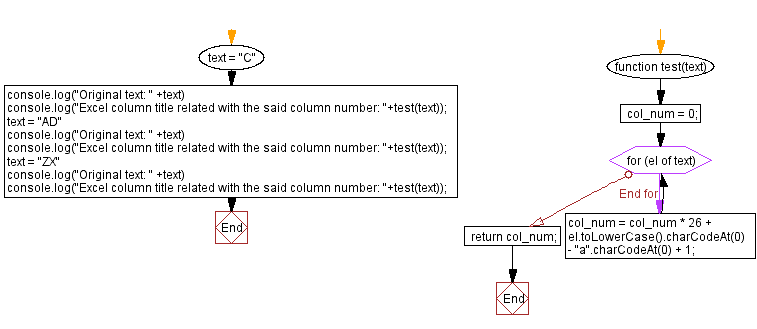
Live Demo:
See the Pen javascript-math-exercise-98-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts an Excel column title to its corresponding number by processing each letter's value.
- Write a JavaScript function that iterates through the characters of a column title and computes the numeric value using base-26 arithmetic.
- Write a JavaScript function that validates the input title and handles both uppercase and lowercase letters.
- Write a JavaScript function that returns the Excel column number and manages edge cases such as invalid characters.
Improve this sample solution and post your code through Disqus.
Previous: Excel column title related with a column number.
Next: Add digits until there is only one digit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.