JavaScript: Excel column title related with a column number
JavaScript Math: Exercise-97 with Solution
Excel Column Title from Number
Write a JavaScript program to retrieve the Excel column title associated with a given column number (integer value).
For example:
1 -> A
2 -> B
3 -> C
...
26 -> Z
27 -> AA
28 -> AB
...
Test Data:
(3) -> C
(27) -> AA
( 151) -> EU
Sample Solution-1:
JavaScript Code:
/**
* Function to convert a column number to its corresponding Excel column title.
* @param {number} n - The column number.
* @returns {string} - The Excel column title.
*/
function test(n) {
s = 1; // Start index for column titles (A = 1)
e = 26; // End index for column titles (Z = 26)
result = ""; // Initialize the result variable to store the column title
while ((n -= s) >= 0) { // Loop until the column number is greater than or equal to the start index
result = String.fromCharCode(parseInt((n % e) / s) + 65) + result; // Calculate the character for the current digit and prepend it to the result
s = e; // Update the start index for the next digit
e *= 26; // Update the end index for the next digit
}
return result; // Return the Excel column title
}
// Test cases
let n = 4;
console.log("n = " + n);
console.log("Excel column title related with the said column number: " + test(n));
n = 27;
console.log("n = " + n);
console.log("Excel column title related with the said column number: " + test(n));
n = 151;
console.log("n = " + n);
console.log("Excel column title related with the said column number: " + test(n));
Output:
n = 4 Excel column title related with the said column number: D n = 27 Excel column title related with the said column number: AA n = 151 Excel column title related with the said column number: EU
Flowchart:
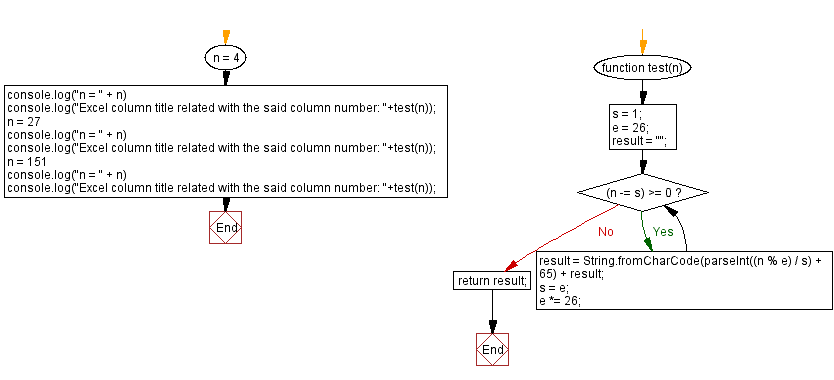
Live Demo:
See the Pen javascript-math-exercise-97 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
/**
* Function to convert a column number to its corresponding Excel column title.
* @param {number} n - The column number.
* @returns {string} - The Excel column title.
*/
function test(n) {
result = ''; // Initialize the result variable to store the Excel column title
while(n > 0){ // Loop until n becomes 0
el = String.fromCharCode(65 + (n-1) % 26); // Calculate the character corresponding to the current digit
result = el + result; // Prepend the character to the result
n = ~~((n-1)/26); // Update n to get the next digit
}
return result; // Return the Excel column title
}
// Test cases
n = 4;
console.log("n = " + n);
console.log("Excel column title related with the said column number: " + test(n));
n = 27;
console.log("n = " + n);
console.log("Excel column title related with the said column number: " + test(n));
n = 151;
console.log("n = " + n);
console.log("Excel column title related with the said column number: " + test(n));
Output:
n = 4 Excel column title related with the said column number: D n = 27 Excel column title related with the said column number: AA n = 151 Excel column title related with the said column number: EU
Flowchart:
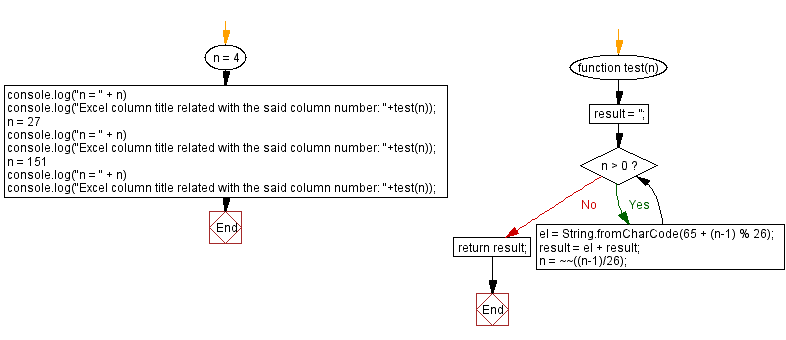
Live Demo:
See the Pen javascript-math-exercise-97-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a given positive integer to its corresponding Excel column title using modulus and division.
- Write a JavaScript function that iteratively builds the Excel column title by mapping remainders to letters.
- Write a JavaScript function that validates the input and returns an error if the column number is not a positive integer.
- Write a JavaScript function that handles edge cases where the column number requires multiple letters, such as 27 or above.
Improve this sample solution and post your code through Disqus.
Previous: Number of trailing zeroes in a factorial.
Next: Column number that relates to a column title.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.