JavaScript: Number of trailing zeroes in a factorial
JavaScript Math: Exercise-96 with Solution
Factorial Trailing Zeroes
Write a JavaScript program that calculates the factorial of a number and returns the number of trailing zeroes.
In mathematics, the factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n. The factorial of n also equals the product of n with the next smaller factorial:
n! = n x (n-1) x (n-2) x (n-3)x.....x 3 x 2 x 1
= n x (n-1)!
For example 4! = 4 x 3! = 4 x 3 x 2 x 1 =24
The value of 0! is 1, according to the convention for an empty product.
Test Data:
(4) -> 0
(9) -> 1
(10) -> 2
(23) -> 4
(35) -> 8
Sample Solution-1:
JavaScript Code:
/**
* Function to count the number of trailing zeroes in the factorial of a given number.
* @param {number} n - The input number.
* @returns {number} - The count of trailing zeroes.
*/
function test(n) {
ctr = 0; // Initialize the counter for trailing zeroes
i = 5; // Initialize the divisor
while (n / i >= 1) {
ctr += Math.floor(n / i); // Increment the counter based on the quotient
i *= 5; // Update the divisor for the next iteration
}
return ctr; // Return the count of trailing zeroes
}
// Test cases
let n = 4;
console.log("n = " + n);
console.log("Number of trailing zeroes in the factorial: " + test(n));
n = 9;
console.log("n = " + n);
console.log("Number of trailing zeroes in the factorial: " + test(n));
n = 10;
console.log("n = " + n);
console.log("Number of trailing zeroes in the factorial: " + test(n));
n = 23;
console.log("n = " + n);
console.log("Number of trailing zeroes in the factorial: " + test(n));
n = 35;
console.log("n = " + n);
console.log("Number of trailing zeroes in the factorial: " + test(n));
Output:
n = 4 Number of trailing zeroes in the said factorial: 0 n = 9 Number of trailing zeroes in the said factorial: 1 n = 10 Number of trailing zeroes in the said factorial: 2 n = 23 Number of trailing zeroes in the said factorial: 4 n = 35 Number of trailing zeroes in the said factorial: 8
Flowchart:
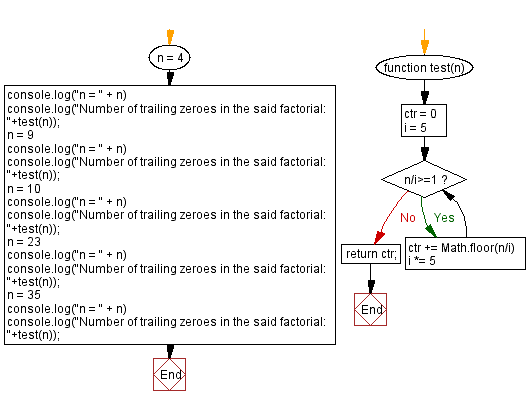
Live Demo:
See the Pen javascript-math-exercise-96 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
/**
* Function to count the number of trailing zeroes in the factorial of a given number.
* @param {number} n - The input number.
* @returns {number} - The count of trailing zeroes.
*/
function test(n) {
pow = Math.log(n) / Math.log(5); // Calculate the maximum power of 5 that divides n
result = 0; // Initialize the result variable
for (i = 1; i <= pow; i++) { // Iterate from 1 to the calculated power
result += Math.floor(n / Math.pow(5, i)); // Update the result by summing up the quotients of n divided by powers of 5
};
return result; // Return the count of trailing zeroes
}
// Test cases
let n = 4;
console.log("n = " + n);
console.log("Number of trailing zeroes in the said factorial: " + test(n));
n = 9;
console.log("n = " + n);
console.log("Number of trailing zeroes in the said factorial: " + test(n));
n = 10;
console.log("n = " + n);
console.log("Number of trailing zeroes in the said factorial: " + test(n));
n = 23;
console.log("n = " + n);
console.log("Number of trailing zeroes in the said factorial: " + test(n));
n = 35;
console.log("n = " + n);
console.log("Number of trailing zeroes in the said factorial: " + test(n));
Output:
n = 4 Number of trailing zeroes in the said factorial: 0 n = 9 Number of trailing zeroes in the said factorial: 1 n = 10 Number of trailing zeroes in the said factorial: 2 n = 23 Number of trailing zeroes in the said factorial: 4 n = 35 Number of trailing zeroes in the said factorial: 8
Flowchart:
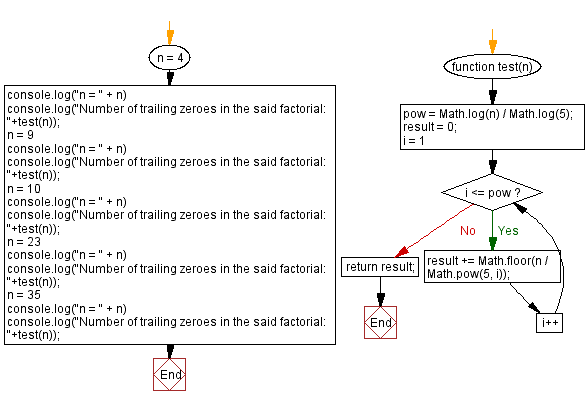
Live Demo:
See the Pen javascript-math-exercise-96-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the factorial of a number indirectly by counting factors of 5 to determine trailing zeroes.
- Write a JavaScript function that calculates trailing zeroes in n! without computing the full factorial using iterative division.
- Write a JavaScript function that uses a loop to divide the number by 5 repeatedly and sums the quotients to find the zero count.
- Write a JavaScript function that handles large input values efficiently when calculating the number of trailing zeroes.
Improve this sample solution and post your code through Disqus.
Previous: Last and middle character from a string.
Next: Excel column title related with a column number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.