JavaScript: Last and middle character from a string
JavaScript Math: Exercise-95 with Solution
Last or Middle Character of Words
Write a JavaScript program that takes text and returns the last character of each word if it is even in length. It also returns the middle character if it is odd in length.
Test Data:
("Number of even numbers and their sum") -> "r, f, n, b, n, e, u"
("JavaScript Math and Numbers- Exercises Practice Solution") -> "t, h, n, -, c, e, n"
Sample Solution:
JavaScript Code:
/**
* Function to process a string and return the last character of even-length words
* or the middle character of odd-length words.
* @param {string} text - The input string to be processed.
* @returns {string} - The result string containing selected characters from each word.
*/
function test(text) {
return text
.split(" ") // Split the input string into an array of words
.map((word) =>
!(word.length % 2) ? word[word.length - 1] : word[Math.floor(word.length / 2)]
) // Map each word to its selected character based on its length
.join(", "); // Join the characters into a single string with commas
}
// Test cases
let text = "Number of even numbers and their sum";
console.log("Original string: " + text);
let result = test(text);
console.log("Processed string: " + result);
text = "JavaScript Math and Numbers- Exercises Practice Solution";
console.log("Original string: " + text);
result = test(text);
console.log("Number of even numbers and their sum: "+result);
Output:
Original string: Number of even numbers and their sum Number of even numbers and their sum: r, f, n, b, n, e, u Original string: JavaScript Math and Numbers- Exercises Practice Solution Number of even numbers and their sum: t, h, n, -, c, e, n
Flowchart:
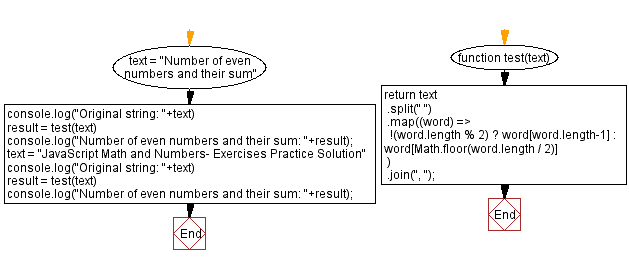
Live Demo:
See the Pen javascript-math-exercise-95 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that processes a sentence and returns the last character of each even-length word and the middle character of each odd-length word.
- Write a JavaScript function that splits a string into words and conditionally extracts either the last or middle character based on word length.
- Write a JavaScript function that handles punctuation and extra spaces while determining the appropriate character from each word.
- Write a JavaScript function that outputs the extracted characters as a comma-separated string.
Improve this sample solution and post your code through Disqus.
Previous: Even, odd numbers and their sum in an array.
Next: Number of trailing zeroes in a factorial.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.