JavaScript: Check downward trend, array of integers
JavaScript Math: Exercise-93 with Solution
Check Downward Trend in Array
Write a JavaScript program to check if an array of integers has a downward trend or not.
Test Data:
([1, 3, 4, 7, 9, 10, 11]) -> false
([11, 10, 9, 7, 4, 3, 2, 0]) -> true
([1, 0, -2, -3, -12]) -> true
Sample Solution:
JavaScript Code:
/**
* Function to check if the elements in the array are in descending order.
* @param {number[]} nums - The array to be checked.
* @returns {boolean} - Returns true if the array is in descending order, false otherwise.
*/
function test(nums) {
return nums.every((item, i) => item > nums[i + 1] || item === nums[nums.length - 1]);
}
// Test cases
let nums = [1, 3, 4, 7, 9, 10, 11];
console.log("n = " + nums);
console.log("Process: " + test(nums));
nums = [11, 10, 9, 7, 4, 3, 2, 0];
console.log("n = " + nums);
console.log("Process: " + test(nums));
nums = [1, 0, -2, -3, -12];
console.log("n = " + nums);
console.log("Process: " + test(nums));
Output:
n = 1,3,4,7,9,10,11 process: false n = 11,10,9,7,4,3,2,0 process: true n = 1,0,-2,-3,-12 process: true
Flowchart:
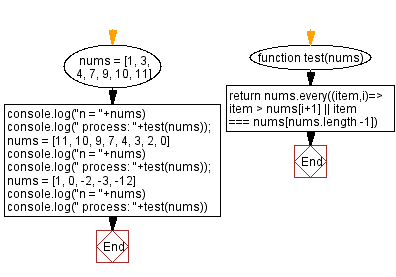
Live Demo:
See the Pen javascript-math-exercise-93 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if an array of integers is strictly decreasing by comparing each element to the next.
- Write a JavaScript function that uses the every() method to verify that every element is greater than its successor.
- Write a JavaScript function that iterates over an array and returns true if a downward trend is detected.
- Write a JavaScript function that handles arrays with a single element or empty arrays appropriately when checking for trends.
Improve this sample solution and post your code through Disqus.
Previous: Iterated Cube Root.
Next: Even, odd numbers and their sum in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics