JavaScript: Test if a number is a Harshad Number or not
JavaScript Math: Exercise-90 with Solution
Check Harshad Number
Write a JavaScript program to check whether a number is a Harshad Number or not.
In recreational mathematics, a harshad number in a given number base, is an integer that is divisible by the sum of its digits when written in that base.
Example: Number 200 is a Harshad Number because the sum of digits 2 and 0 and 0 is 2 (2+0+0) and 200 is divisible by 2. Number 171 is a Harshad Number because the sum of digits 1 and 7 and 1 is 9(1+7+1) and 171 is divisible by 9.
Test Data:
(113) -> false
(171) -> true
(200) -> true
Sample Solution:
JavaScript Code:
/**
* Function to check if a number is a Harshad number or not.
* @param {number} n - The number to be checked.
* @returns {boolean} - True if the number is a Harshad number, false otherwise.
*/
function test(n) {
// Convert the number to a string
n = n.toString();
// Initialize the total sum of digits
var total = 0;
// Loop through each digit of the number
for (var i = 0; i < n.length; i++) {
// Add each digit to the total sum
total += parseInt(n[i]);
}
// Convert the number back to an integer
n = parseInt(n);
// Check if the number is divisible by the total sum of its digits
if (n % total === 0) {
// If divisible, return true
return true;
}
// If not divisible, return false
return false;
}
// Test cases
// Test if the number 113 is a Harshad number
n = 113;
console.log("n = " + n);
console.log("Test the said number is Harshad Number or not! " + test(n));
// Test if the number 171 is a Harshad number
n = 171;
console.log("n = " + n);
console.log("Test the said number is Harshad Number or not! " + test(n));
// Test if the number 200 is a Harshad number
n = 200;
console.log("n = " + n);
console.log("Test the said number is Harshad Number or not! " + test(n));
Output:
n = 113 Test the said number is Harshad Number or not! false n = 171 Test the said number is Harshad Number or not! true n = 200 Test the said number is Harshad Number or not! true
Flowchart:
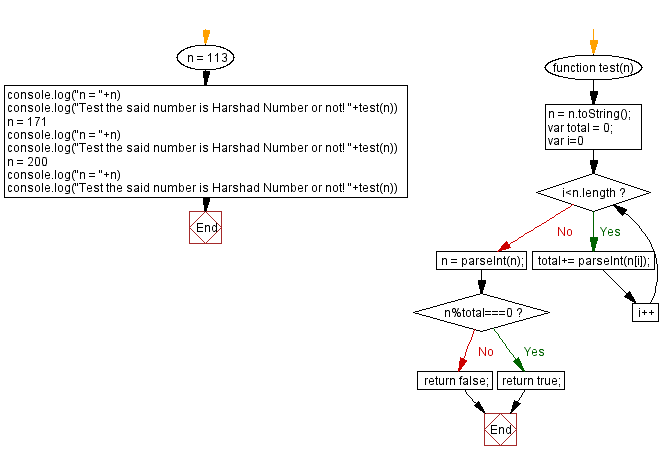
Live Demo:
See the Pen javascript-math-exercise-90 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that determines if a number is a Harshad number by summing its digits and checking divisibility.
- Write a JavaScript function that converts a number to a string, computes the digit sum, and tests divisibility.
- Write a JavaScript function that handles edge cases such as zero and negative numbers when checking for Harshad numbers.
- Write a JavaScript function that returns a boolean indicating if a number is Harshad and logs the intermediate digit sum.
Improve this sample solution and post your code through Disqus.
Previous: Sum of oddly divisible numbers from a range.
Next: Sum of all odds in a matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.