JavaScript: Sum of oddly divisible numbers from a range
JavaScript Math: Exercise-89 with Solution
Oddly Divisible Sum in Range
Write a JavaScript program that takes three arguments x, y, n and calculates the sum of the numbers oddly divided by n from the range x, y inclusive.
Test Data:
(1,5,3) -> 3
(100, 1000, 5) -> 99550
Sample Solution:
JavaScript Code:
/**
* Function to calculate the sum of numbers divisible by a given number within a specified range.
* @param {number} x - The starting value of the range.
* @param {number} y - The ending value of the range.
* @param {number} n - The divisor to check divisibility.
* @returns {number} - The sum of numbers divisible by 'n' within the range from 'x' to 'y'.
*/
function test(x, y, n) {
// Initialize the sum of divisible numbers
let num_sum = 0;
// Loop through each number within the specified range
for (let i = x; i <= y; i++) {
// If the current number is divisible by 'n', add it to the sum; otherwise, add 0
num_sum += i % n === 0 ? i : 0;
}
// Return the sum of divisible numbers
return num_sum;
}
// Test cases
// Define the range and divisor for the first test case
x = 1;
y = 5;
n = 3;
// Print range and divisor information
console.log("Range: " + x + "-" + y);
console.log("Divisible number: " + n);
// Calculate and print the sum of oddly divisible numbers within the specified range
console.log("Sum of oddly divisible numbers from the said range: " + test(x, y, n));
// Repeat the above steps for a different range and divisor
x = 100;
y = 1000;
n = 5;
console.log("Range: " + x + "-" + y);
console.log("Divisible number: " + n);
console.log("Sum of oddly divisible numbers from the said range: " + test(x, y, n));
Output:
Range: 1-5 Divisible number: 3 Sum of oddly divisible numbers from a the said range: 3 Range: 100-1000 Divisible number: 5 Sum of oddly divisible numbers from a the said range: 99550
Flowchart:
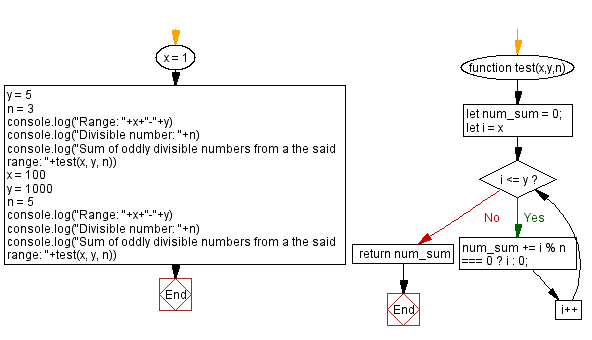
Live Demo:
See the Pen javascript-math-exercise-89 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that sums numbers in a range that, when divided by a given divisor, yield an odd remainder.
- Write a JavaScript function that filters numbers within a range based on an odd remainder criterion and then computes their sum.
- Write a JavaScript function that iterates through a numerical range, checks for odd divisibility, and accumulates the total.
- Write a JavaScript function that validates the range and divisor before calculating the sum of oddly divisible numbers.
Improve this sample solution and post your code through Disqus.
Previous: Check to given vectors are orthogonal or not.
Next: Test if a number is a Harshad Number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.