JavaScript: Check to given vectors are orthogonal or not
JavaScript Math: Exercise-88 with Solution
Write a JavaScript program to check whether two vectors are orthogonal or not.
Two vectors are orthogonal if they are perpendicular to each other. i.e. the dot product of the two vectors is zero.
Let u and v are two vectors. The vectors u, v will be orthogonal if they are perpendicular, i.e., they form a right angle, or if the dot product they yield is zero.
u⊥v or u•v=0
Visualisation:
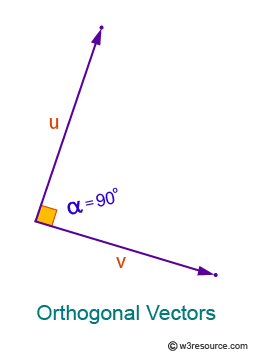
Test Data:
([2, 4, 1], [2, 1, -8]) -> true
([1, 0, 1], [0, 0, 1]) -> false
([1, 0, 0], [0, 1, 0]) -> true
Sample Solution:
JavaScript Code:
/**
* Function to check if two vectors are orthogonal or not.
* @param {number[]} nums1 - The first vector.
* @param {number[]} nums2 - The second vector.
* @returns {boolean} - True if the vectors are orthogonal, false otherwise.
*/
function test(nums1, nums2) {
// Initialize total sum to 0
var total = 0;
// Iterate through the elements of the vectors
for(var i=0;i<nums1.length;i++){
// Add the product of corresponding elements to the total sum
total+=(nums1[i] *nums2[i]);
}
// Check if the total sum is zero (indicating orthogonality)
return total == 0;
}
// Test cases
// Define the vectors
nums1 = [2, 4, 1];
nums2 = [2, 1, -8];
// Print the original vectors
console.log("Original vectors:");
console.log(nums1+"\n"+nums2);
// Check if the vectors are orthogonal and print the result
console.log("Check the said vectors are orthogonal or not: " + test(nums1, nums2));
// Repeat the above steps for different vectors
nums1 = [1, 0, 1];
nums2 = [0, 0, 1];
console.log("Original vectors:");
console.log(nums1+"\n"+nums2);
console.log("Check the said vectors are orthogonal or not: " + test(nums1, nums2));
nums1 = [1, 0, 0];
nums2 = [0, 1, 0];
console.log("Original vectors:");
console.log(nums1+"\n"+nums2);
console.log("Check the said vectors are orthogonal or not: " + test(nums1, nums2));
Output:
Original vectors: 2,4,1 2,1,-8 Check the said vectors are orthogonal or not: true Original vectors: 1,0,1 0,0,1 Check the said vectors are orthogonal or not: false Original vectors: 1,0,0 0,1,0 Check the said vectors are orthogonal or not: true
Flowchart:
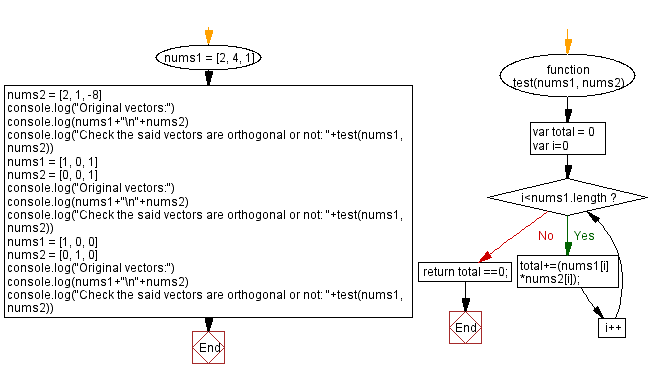
Live Demo:
See the Pen javascript-math-exercise-88 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Check a number is Sastry number or not.
Next: Sum of oddly divisible numbers from a range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-math-exercise-88.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics