JavaScript: Check a number is Sastry number or not
JavaScript Math: Exercise-87 with Solution
Check Sastry Number
Write a JavaScript program that checks whether a number (positive integer) is a Sastry number or not.
The number N is a Sastry number when it is concatenated with N+1 and gives a perfect squares. Some Sastry numbers are 183, 328, 528, 715, 6099, 13224, 40495, 106755, 453288, 2066115, 2975208, 22145328, 28027683, 110213248...
Test Data:
(27) -> false
(328) -> true
(28027683) -> true
Sample Solution:
JavaScript Code:
/**
* Function to check if a number is a Sastry number or not.
* @param {number} n - The input number.
* @returns {boolean} - True if the number is a Sastry number, false otherwise.
*/
function test(n){
// Concatenate n with n+1 and convert it to a string, then find the square root and check if it's an integer
return Number.isInteger(Math.sqrt(`${n}${n + 1}`));
}
// Test cases
// Assign value to n
n = 27;
// Print the original number
console.log("Original number: " + n);
// Check if the number is a Sastry number and print the result
console.log("Check the said number is Sastry number or not: " + test(n));
// Repeat the above steps for different values of n
n = 328;
console.log("Original number: " + n);
console.log("Check the said number is Sastry number or not: " + test(n));
n = 28027683;
console.log("Original number: " + n);
console.log("Check the said number is Sastry number or not: " + test(n));
Output:
Original string: 27 Check the said number is Sastry number or not: false Original string: 328 Check the said number is Sastry number or not: true Original string: 28027683 Check the said number is Sastry number or not: true
Flowchart:
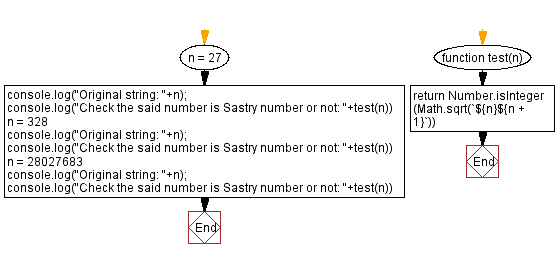
Live Demo:
See the Pen javascript-math-exercise-87 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a number is Sastry by concatenating it with its successor and testing if the result is a perfect square.
- Write a JavaScript function that computes the concatenated number of N and N+1, then determines if it is a perfect square.
- Write a JavaScript function that validates the input and handles non-integer values when checking for Sastry numbers.
- Write a JavaScript function that optimizes the Sastry number test by using integer arithmetic to approximate the square root.
Improve this sample solution and post your code through Disqus.
Previous: Middle character(s) of a string.
Next: Check to given vectors are orthogonal or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics