JavaScript: Middle character(s) of a string
JavaScript Math: Exercise-86 with Solution
Get Middle Character(s) of String
Write a JavaScript program to get the middle character(s) from a given string.
Test Data:
("abcd") -> "bc"
("abc") -> "b"
("JavaScript") -> "Sc"
Sample Solution:
JavaScript Code:
/**
* Function to find the middle character(s) of a given string.
* @param {string} text - The input string.
* @returns {string} - The middle character(s) of the string.
*/
function test(text) {
// Get the length of the input string
var text_len = text.length;
// Check if the length of the string is odd or even
if (text_len % 2 != 0) {
// Calculate the start index for odd-length strings
let start = (text_len - 1) / 2;
// Return the middle character
return text.slice(start, start + 1);
} else {
// Calculate the start index for even-length strings
let start = text_len / 2 - 1;
// Return the middle two characters for even-length strings
return text.slice(start, start + 2);
}
}
// Test cases
// Assign value to text
text = "abcd";
// Print the original string
console.log("Original string: " + text);
// Print the middle character(s) of the said string
console.log("Middle character(s) of the said string: " + test(text));
// Repeat the above steps for different values of text
text = "abc";
console.log("Original string: " + text);
console.log("Middle character(s) of the said string: " + test(text));
text = "JavaScript";
console.log("Original string: " + text);
console.log("Middle character(s) of the said string: " + test(text));
Output:
Original string: abcd Middle character(s) of the said string: bc Original string: abc Middle character(s) of the said string: b Original string: JavaScript Middle character(s) of the said string: Sc
Flowchart:
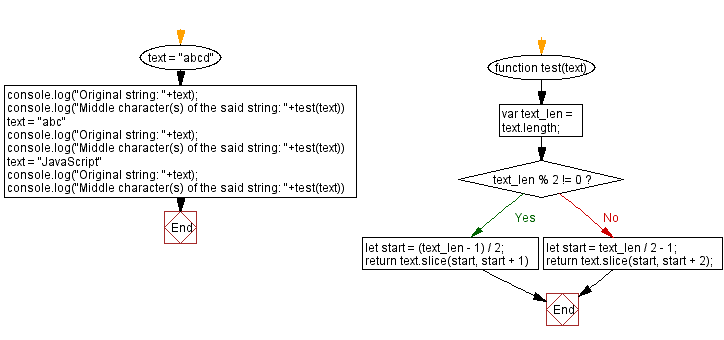
Live Demo:
See the Pen javascript-math-exercise-86 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the middle character(s) of a string depending on whether its length is odd or even.
- Write a JavaScript function that calculates the correct starting index and length to extract the middle part of a string.
- Write a JavaScript function that handles edge cases like empty strings or single-character strings when determining the middle characters.
- Write a JavaScript function that returns the middle characters in uppercase for consistency in output.
Go to:
PREV : Sum of Main Diagonal Elements in Matrix.
NEXT : Check Sastry Number.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.