JavaScript: Sum of the main diagonal elements of a square matrix
JavaScript Math: Exercise-85 with Solution
Sum of Main Diagonal Elements in Matrix
Write a JavaScript program to compute the sum of the main diagonal elements of a square matrix.
In mathematics, a matrix (plural matrices) is a rectangular array or table of numbers, symbols, or expressions, arranged in rows and columns, which is used to represent a mathematical object or a property of such an object.
An m × n matrix: the m rows are horizontal and the n columns are vertical
In mathematics, a square matrix is a matrix with the same number of rows and columns. An n-by-n matrix is known as a square matrix of order n. Any two square matrices of the same order can be added and multiplied.
Example:
Test Data:
([ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]) -> 15
( [ [-1, -2], [-4, -5] ]) -> -6
Sample Solution:
JavaScript Code:
/**
* Function to find the sum of the main diagonal elements of a square matrix.
* @param {number[][]} nums - The input square matrix.
* @returns {number} - The sum of the main diagonal elements.
*/
function test(nums) {
// Use reduce to sum the elements along the main diagonal of the matrix
return nums.reduce((x, y, i) => x + y[i], 0);
}
// Test cases
// Assign value to nums
nums = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
// Print the sum of the main diagonal elements of the said square matrix
console.log("Sum of the main diagonal elements of the said square matrix: " + test(nums));
// Repeat the above steps for a different value of nums
nums = [
[-1, -2],
[-4, -5]
];
console.log("Sum of the main diagonal elements of the said square matrix: " + test(nums));
Output:
Sum of the main diagonal elements of the said square matrix: 15 Sum of the main diagonal elements of the said square matrix: -6
Flowchart:
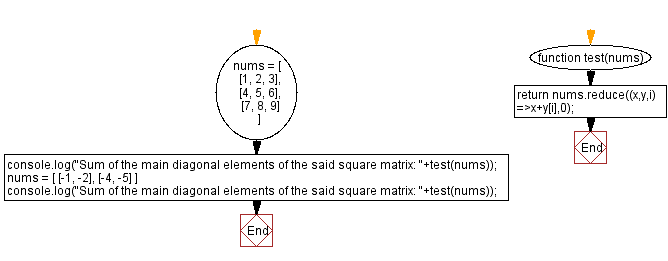
Live Demo:
See the Pen javascript-math-exercise-85 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the sum of the main diagonal elements of a square matrix using a for loop.
- Write a JavaScript function that uses the reduce() method on the matrix rows to calculate the diagonal sum.
- Write a JavaScript function that validates that the input is a square matrix before summing its diagonal elements.
- Write a JavaScript function that iterates over a matrix and sums elements where the row index equals the column index.
Improve this sample solution and post your code through Disqus.
Previous: Sum of the Two highest Numbers.
Next: Middle character(s) of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.