JavaScript: Missing number from an array
JavaScript Math: Exercise-83 with Solution
Write a JavaScript program to find the missing number in a given array. There are no duplicates in the list.
Test Data:
([1,2,3,5,6,7]) -> 4
([2,3,4,5]) -> 1
Sample Solution:
JavaScript Code:
/**
* Function to find the missing number in an array of consecutive integers.
* @param {number[]} nums - The input array of consecutive integers.
* @returns {number} - The missing number in the array.
*/
function test(nums) {
// Iterate over consecutive integers up to the length of the input array + 1
for (let n = 1; n <= nums.length + 1; n++) {
// Check if the current integer is not found in the input array
if (nums.indexOf(n) === -1)
// If the current integer is not found, return it as the missing number
return n;
}
}
// Test cases
// Assign value to nums
nums = [1, 2, 3, 5, 6, 7];
// Print the value of nums
console.log("nums = " + nums);
// Find the missing number in the said array
console.log("Missing number of the said array: " + test(nums));
// Repeat the above steps for a different value of nums
nums = [2, 3, 4, 5];
console.log("nums = " + nums);
console.log("Missing number of the said array: " + test(nums));
Output:
nums = 1,2,3,5,6,7 Missing number of the said array: 4 nums= 2,3,4,5 Missing number of the said array: 1
Flowchart:
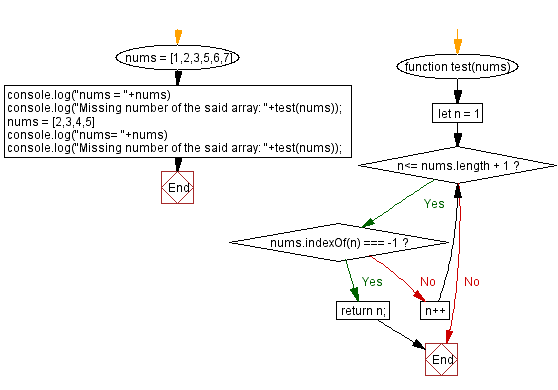
Live Demo:
See the Pen javascript-math-exercise-83 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Mean of all digits of a number.
Next: Sum of the Two highest Numbers
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-math-exercise-83.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics