JavaScript: Mean of all digits of a number
JavaScript Math: Exercise-82 with Solution
Mean of Digits in a Number
Write a JavaScript program to calculate the mean of all the digits of a given number.
What is a Mean?
In mathematics, the mean represents the simple average of two or more numbers. There are several methods available for computing the mean of a set of numbers, including the arithmetic mean method, which uses the sum of the numbers in the series, and the geometric mean method, which is the average of a set of products.
Test Data:
(11) -> 1
(66) -> 6
(336) -> 4
(444) -> 4
(1151) -> 2
Sample Solution:
JavaScript Code:
/**
* Function to calculate the mean of all digits of a number.
* @param {number} n - The input number.
* @returns {number} - The mean of all digits of the input number.
*/
function test(n) {
// Convert the absolute value of n to a string and split it into an array of characters
let arr_str = String(Math.abs(n)).split('');
// Reduce the array of characters to the sum of their numeric values and divide by the number of digits
return arr_str.reduce((a, b) => a + Number(b), 0) / arr_str.length;
}
// Test cases
// Assign value to n
n = 11;
// Print the value of n
console.log("n = " + n);
// Calculate the mean of all digits of the said number
console.log("Mean of all digits of the said number: " + test(n));
// Repeat the above steps for different values of n
n = 66;
console.log("n = " + n);
console.log("Mean of all digits of the said number: " + test(n));
n = 336;
console.log("n = " + n);
console.log("Mean of all digits of the said number: " + test(n));
n = 444;
console.log("n = " + n);
console.log("Mean of all digits of the said number: " + test(n));
n = 1151;
console.log("n = " + n);
console.log("Mean of all digits of the said number: " + test(n));
Output:
n = 11 Mean of all digits of the said number: 1 n = 66 Mean of all digits of the said number: 6 n = 336 Mean of all digits of the said number: 4 n = 444 Mean of all digits of the said number: 4 n = 1151 Mean of all digits of the said number: 2
Flowchart:
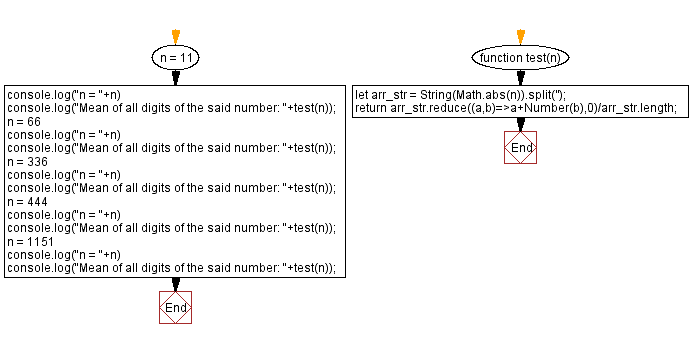
Live Demo:
See the Pen javascript-math-exercise-82 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the arithmetic mean of the digits of a given number by summing and counting the digits.
- Write a JavaScript function that converts a number to a string, computes the mean of its digits, and returns the result.
- Write a JavaScript function that handles negative numbers by using the absolute value before calculating the mean.
- Write a JavaScript function that returns an object containing both the mean and the total digit count from a number.
Improve this sample solution and post your code through Disqus.
Previous: Multiply every digit of a number three times.
Next: Missing number from an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.