JavaScript: Check an integer is Repdigit number or not
JavaScript Math: Exercise-80 with Solution
Check Repdigit Number
Write a JavaScript program to check whether a given integer is a Repdigit or not.
From Wikipedia -In recreational mathematics, a repdigit or sometimes monodigit is a natural number composed of repeated instances of the same digit in a positional number system (often implicitly decimal). The word is a portmanteau of repeated and digit. Examples are 11, 666, 4444, and 999999.
Test Data:
(11) -> true
(66) -> true
(336) -> false
(444) -> true
(1151) -> false
Sample Solution:
JavaScript Code:
/**
* Function to check if a number is a Repdigit.
* @param {number} n - The input number.
* @returns {boolean} - True if the number is a Repdigit, otherwise false.
*/
function test(n) {
// Convert the number to a string and create a set to count unique digits
return new Set('' + n).size === 1;
}
// Test cases
// Assign value to n
n = 11;
// Print the value of n
console.log("n = " + n);
// Check if the said number is Repdigit
console.log("Check the said number is Repdigit: " + test(n));
// Repeat the above steps for different values of n
n = 66;
console.log("n = " + n);
console.log("Check the said number is Repdigit: " + test(n));
n = 336;
console.log("n = " + n);
console.log("Check the said number is Repdigit: " + test(n));
n = 444;
console.log("n = " + n);
console.log("Check the said number is Repdigit: " + test(n));
n = 1151;
console.log("n = " + n);
console.log("Check the said number is Repdigit: " + test(n));
Output:
n = 11 Check the said number is Repdigit: true n = 66 Check the said number is Repdigit: true n = 336 Check the said number is Repdigit: false n = 444 Check the said number is Repdigit: true n = 1151 Check the said number is Repdigit: false
Flowchart:
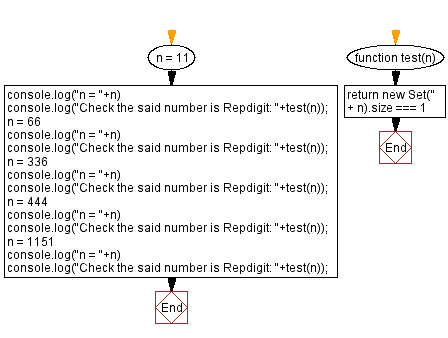
Live Demo:
See the Pen javascript-math-exercise-80 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks whether a number is a repdigit by converting it to a string and comparing all digits.
- Write a JavaScript function that iterates through the digits of a number and verifies if all digits match the first digit.
- Write a JavaScript function that uses a regular expression to test if a number consists of repeated digits.
- Write a JavaScript function that handles single-digit numbers and returns true as they are trivially repdigits.
Improve this sample solution and post your code through Disqus.
Previous: Sequence number from the given Triangular Number.
Next: Multiply every digit of a number three times.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.